


Why Does Appending Pointers in Go's `append` Function Lead to Unexpected Overwriting?
Unexpected Overwriting Issue with Go's "append" Function
In Go, the append function is frequently used to extend slices with additional elements. However, occasionally, unexpected behavior can occur when appending pointers to structures.
Consider the following code snippet:
import "fmt" type Foo struct { val int } func main() { var a = make([]*Foo, 1) a[0] = &Foo{0} var b = [3]Foo{Foo{1}, Foo{2}, Foo{3}} for _, e := range b { a = append(a, &e) } for _, e := range a { fmt.Printf("%v ", *e) } }
Intriguingly, instead of printing {0} {1} {2} {3}, the code outputs {0} {3} {3} {3}. This unexpected behavior stems from a fundamental aspect of Go's behavior:
Copies in for Range Loops
Go iterates over slices and arrays using for range loops, which create a copy of each element during iteration. In our code, the for range loop variable e represents a temporary copy of the array element b[i]. Unfortunately, we are then appending the address of this temporary variable to the slice a.
The Fix
To resolve this issue, we need to append the address of the original array element instead of the temporary copy. By modifying the for loop to use the index i, we ensure that the correct address is appended to the slice:
for i := range b { a = append(a, &b[i]) }
This change yields the expected output of {0} {1} {2} {3}.
Reason for the Behavior
Go's lack of true references contributes to this behavior. In C or Java, where references are used, the loop variable would directly reference the array element, allowing mutations via the loop variable. However, in Go, the loop variable is a distinct variable that holds a copy of the element, not a reference. Consequently, changes made to the loop variable are not reflected in the original array elements.
The above is the detailed content of Why Does Appending Pointers in Go's `append` Function Lead to Unexpected Overwriting?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


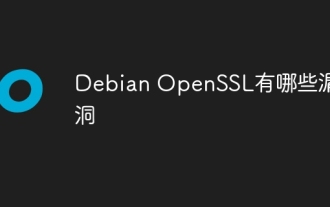
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
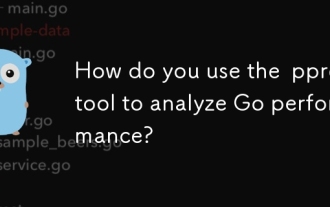
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
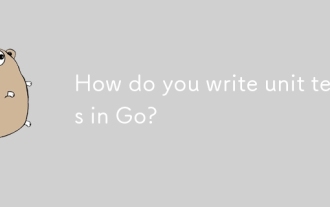
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
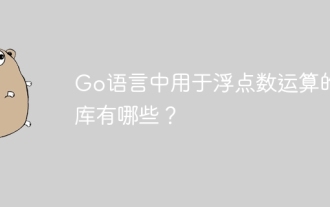
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
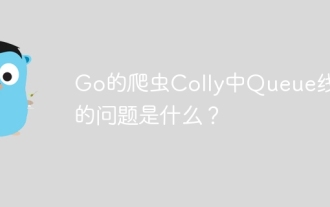
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
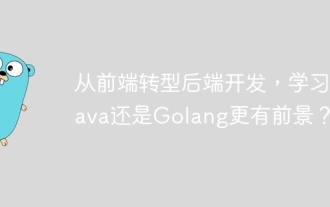
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
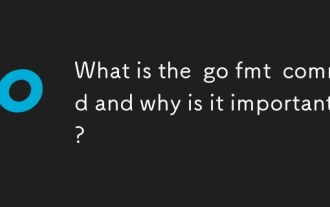
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
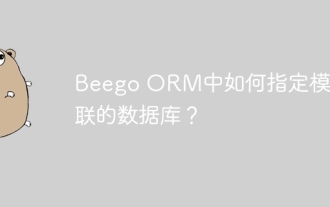
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
