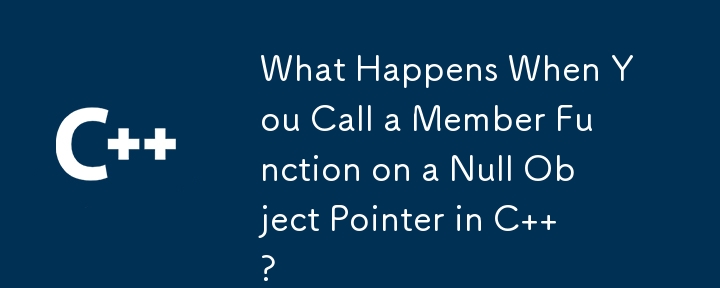
What Happens When a Member Function is Called on a Null Object Pointer?
In C , calling a member function on a null object pointer is considered undefined behavior. This means that the outcome of such an action is unpredictable and may vary across different compilers and platforms.
Consider the following code snippet:
class A
{
public:
void fun()
{
std::cout << "fun" << std::endl;
}
};
A* a = NULL;
a->fun();
Copy after login
When this code is executed, it attempts to call the fun() member function on a null pointer (a), which points to no valid object in memory. The behavior of this code is undefined, and several scenarios may occur:
-
Crash: In some cases, calling a member function on a null pointer may cause a program crash due to an access violation. When the object pointer is dereferenced (e.g., a->fun()), the program may attempt to access an invalid memory location, leading to a runtime error.
-
Segmentation Fault: Similar to a crash, a segmentation fault can occur if the code tries to access memory outside of the valid address space. This can happen when a null pointer is used to access memory that does not belong to the program.
-
Unexpected Output: In some cases, the code may execute without any apparent errors and produce unexpected output. This is because the compiler may generate code that assumes the pointer is not null, even though it actually is. In the provided code, it is possible that the fun() function is executed successfully, printing "fun" to the console.
-
Unpredictable Results: In general, the behavior of calling a member function on a null pointer is unpredictable and may vary depending on factors such as the compiler optimizations, operating system, and hardware architecture. It is not guaranteed to result in a specific outcome and should be avoided.
To prevent undefined behavior, it is crucial to ensure that object pointers are always pointing to valid objects before attempting to call member functions on them. This can be done by checking for null pointers before dereferencing them.
The above is the detailed content of What Happens When You Call a Member Function on a Null Object Pointer in C ?. For more information, please follow other related articles on the PHP Chinese website!