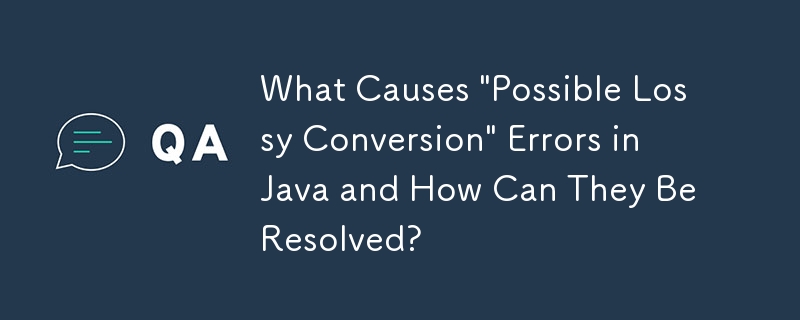
What's the Meaning of "Possible Lossy Conversion"?
Java programmers often encounter compilation errors like "incompatible types: possible lossy conversion from double to int." This error occurs when attempting to convert a numeric value from one primitive type to another with the potential for data loss.
Types Prone to Lossy Conversions
Lossy conversions can happen when converting:
- long to int
- float to long
- double to int, long, or float
Understanding Lossiness
Lossy conversions occur when the converted value cannot be accurately represented in the new type. For example, converting a double (with fractional part) to an int will result in truncation, potentially losing fractional information.
How to Fix "Possible Lossy Conversion"
To resolve this error, you can either:
-
Add a Typecast: Explicitly convert the value to the desired type using typecasting, e.g., int squareRoot = (int) Math.sqrt(i);. However, this may not always be the optimal solution as it can result in data loss.
-
Re-evaluate Code: Identify why the conversion is necessary and consider alternative approaches, such as:
- Changing the variable type to avoid the conversion
- Checking for incorrect/unexpected values and handling them appropriately
- Using methods like rounding to prevent data loss
Possible Lossy Conversions in Specific Contexts
-
Array Subscripting: Attempting to use a floating-point value as an array index can result in a possible lossy conversion since array indexes must be integers.
-
Method/Constructor Calls: Passing an actual parameter of a different type than the formal parameter can also trigger this error.
-
Return Statements: Returning a value of a different type than the method's declared return type requires conversion.
-
Promotion in Expressions: Arithmetic and bitwise operators may promote operands to int or long, leading to potential lossy conversions when assigning the result to a narrower type.
-
Assigning Literals: Assigning numeric literals that are not within the representable range of the target type can trigger a lossy conversion warning.
The above is the detailed content of What Causes 'Possible Lossy Conversion' Errors in Java and How Can They Be Resolved?. For more information, please follow other related articles on the PHP Chinese website!