Can Pointers Be Reassigned Within Go's Struct Pointer Methods?
Pointer Reassignment in Struct Pointer Methods in Go
In Go, when working with structs, it's essential to understand pointer reassignment within struct pointer methods. This is a common question that arises when manipulating and returning pointers.
Can Pointers in Struct Pointer Methods Be Reassigned?
Yes, it's possible to reassign pointers in struct pointer methods in Go. However, there are certain limitations and preferred approaches to consider.
Pointer Manipulation vs. Pointer Interpretation
When working with pointers, it's crucial to distinguish between pointer manipulation and pointer interpretation. Pointer interpretation refers to how the value of a pointer is interpreted, such as whether it points to an integer or a struct. Pointer manipulation, on the other hand, involves modifying the value of the pointer itself.
Receiver Type Limitations
In Go, the receiver type of a struct pointer method cannot be a pointer to a pointer (*T). This means that the method cannot modify the pointer itself but only the pointed object.
Two Approaches to Pointer Reassignment
There are two approaches to reassign pointers in struct pointer methods:
- Passing a Pointer to Pointer: You can write a function (not a method) that takes a pointer to a pointer and modify the pointed object. This approach requires passing the address of the pointer variable.
- Returning the Modified Pointer: You can return the modified pointer from the method, and the caller can assign it to the original pointer variable. This approach avoids the need for passing the address of the pointer and is the preferred approach.
Example with Returning the Modified Pointer
Here's an example of how to reassign a pointer using the second approach:
func (tree *AvlTree) rotateLeftToRoot() { // Do some operations on the AvlTree... if tree == nil { return } prevLeft := tree.left if prevLeft != nil { tree.left = prevLeft.right prevLeft.right = tree tree.updateHeight() // Updating the height of the modified tree prevLeft.updateHeight() // Updating the height of the old tree tree = prevLeft // Reassigning the pointer to the new root } }
Conclusion
While it's possible to reassign pointers in struct pointer methods in Go, there are limitations and preferred approaches to consider. By understanding the difference between pointer manipulation and pointer interpretation, and using the appropriate approaches, you can effectively modify and manipulate pointers in your Go code.
The above is the detailed content of Can Pointers Be Reassigned Within Go's Struct Pointer Methods?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










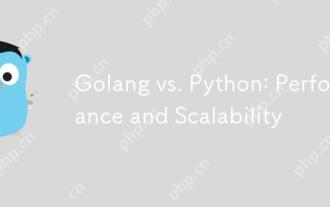
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
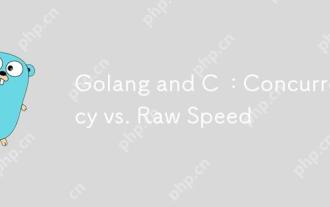
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
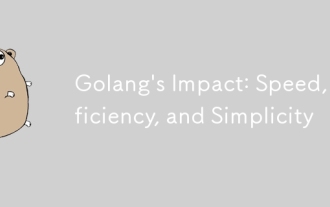
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
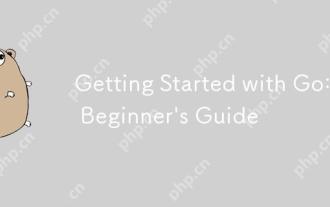
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
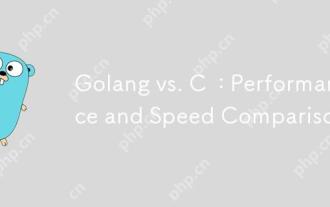
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
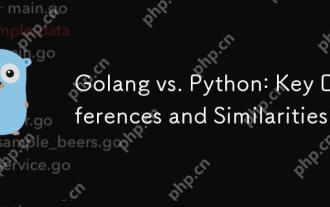
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
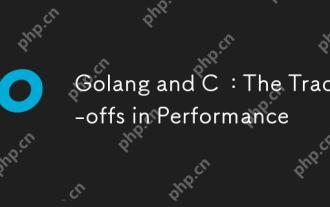
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
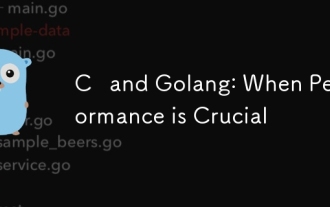
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
