How to Start a Browser After a Go Server Starts Listening?
Handling Browser Startup in Go After Server Listening
In Go, it's possible to encounter a situation where you need to start the browser after the server has started listening. This article provides a solution to tackle this challenge effectively.
Modified Code
The modified code follows a three-step process:
- Open a listener on a specific port.
- Start the browser before entering the server loop.
- Initiate the blocking server loop with http.Serve.
import ( // Standard library packages "fmt" "log" "net" "net/http" // Third party packages "github.com/skratchdot/open-golang/open" "github.com/julienschmidt/httprouter" ) func main() { // Instantiate a new router r := httprouter.New() // Add a handler on /test r.GET("/test", func(w http.ResponseWriter, r *http.Request, _ httprouter.Params) { // Simply write some test data for now fmt.Fprint(w, "Welcome!\n") }) // Open a TCP listener on port 3000 l, err := net.Listen("tcp", "localhost:3000") if err != nil { log.Fatal(err) } // Start the browser to connect to the server err = open.Start("http://localhost:3000/test") if err != nil { log.Println(err) } // Start the blocking server loop log.Fatal(http.Serve(l, r)) }
Explanation
This approach ensures that the browser connects before the server enters the blocking loop in http.Serve. By separating the listening and server loop initiation steps, it allows for browser startup control. The browser can now connect because the listening socket is open.
Conclusion
It's important to note that using ListenAndServe directly skips the socket opening step, resulting in the browser connecting only after the server has started listening. By splitting out these steps, you gain greater control over the browser's startupタイミング and can ensure that it connects at the desired time.
The above is the detailed content of How to Start a Browser After a Go Server Starts Listening?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




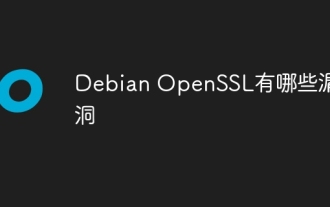
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
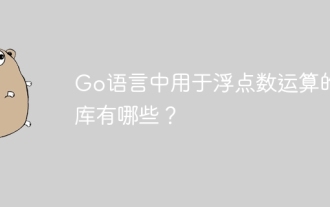
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
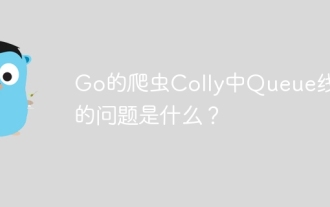
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
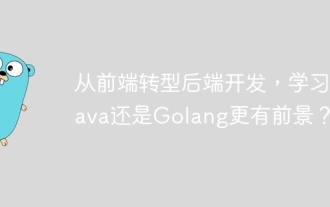
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
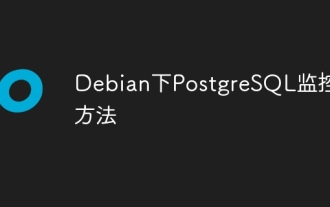
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
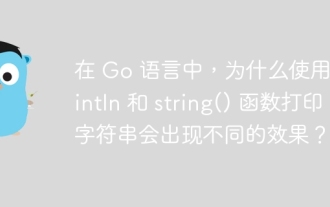
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
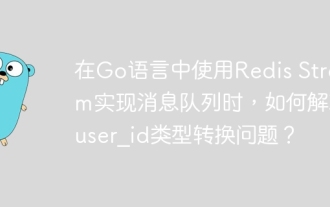
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
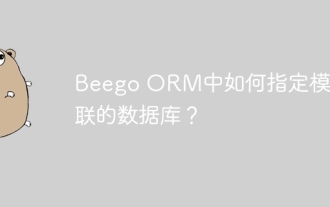
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
