


Curly Braces in ES6 Single Module Imports: When to Use Them and When Not To?
When to Use Curly Braces for ES6 Single Module Imports
In ES6, using curly braces for importing a single module can introduce unexpected behavior. To clarify when to use and avoid curly braces, let's examine the distinction between default and named exports.
Default Export
When importing a single module without curly braces, you're essentially importing the default export. Default exports are declared as:
export default <value>;
In the example provided, initialState.js contains a default export for the initialState object. Therefore, you can access it without curly braces:
import initialState from './todoInitialState';
Named Export
If you need to import a specific exported value, you should use curly braces. Named exports are declared as:
export const <identifier> = <value>;
In the TodoReducer.js example, you're trying to access the todo named export:
import { todo } from './todoInitialState';
Using Curly Braces vs. Avoiding Them
The rule of thumb is to use curly braces when importing named exports and avoid them when importing default exports. Here's a summarized breakdown:
-
Use curly braces:
- When importing a specific named export
-
Avoid curly braces:
- When importing the default export from a module with only one default export
- When importing multiple exports from a module, regardless of whether they're default or named exports
Example
Consider the following example:
// A.js export default MyComponent; export const ChildComponent = () => {};
To import these exports:
-
Default export (MyComponent):
import MyComponent from './A';
Copy after login -
Named export (ChildComponent):
import { ChildComponent } from './A';
Copy after login
By understanding the difference between default and named exports, you can correctly determine when to use curly braces for single module imports in ES6.
The above is the detailed content of Curly Braces in ES6 Single Module Imports: When to Use Them and When Not To?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


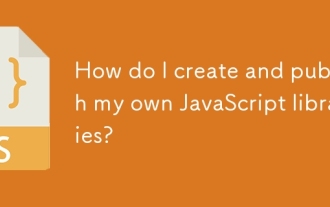
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
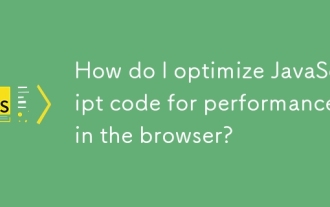
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
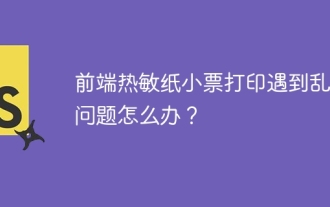
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
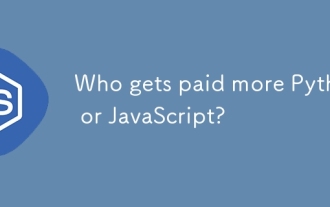
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
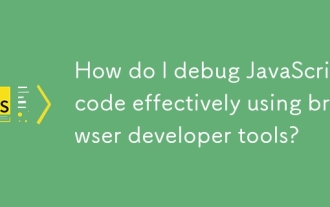
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
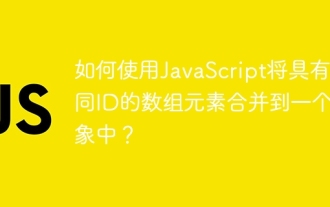
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
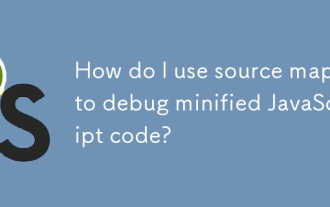
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
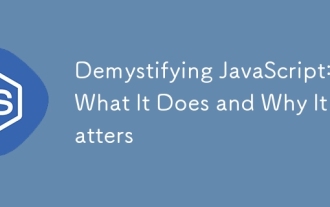
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
