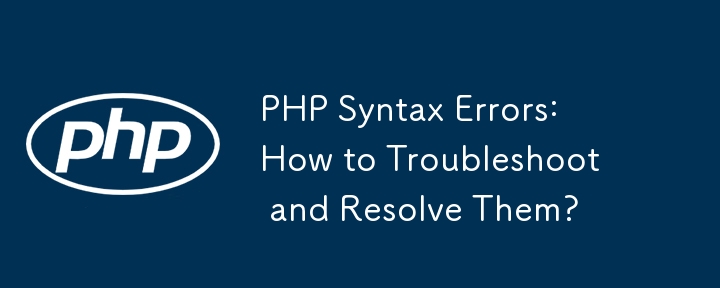
PHP syntax error: how to fix it?
What is the grammatical error?
PHP is a C-style imperative programming language. It has strict syntax rules and cannot recover when it encounters a symbol or identifier in the wrong position. It cannot guess your programming intentions.
Top Tips
There are some basic precautions you can always take:
- Use proper code indentation, Or adopt any good coding style. Readability helps prevent exceptions.
- Use a PHP IDE or editor with syntax highlighting . It also helps with bracket/bracket balance.
- Read carefully the language references and examples in the manual. Read it twice to gain some level of mastery.
How to interpret parser errors
A typical syntax error message is as follows:
Parse error: Syntax error, unexpected in file.php line 217 T_STRING, requires ';'
which lists possible locations of syntax errors. See mentioned File name and Line number. A header like
T_STRING explains which symbol the parser/tokenizer ultimately failed to handle. However, this is not necessarily the cause of the syntax error.
It is important to also look at the lines of code that precede . Often, grammatical errors are just minor mistakes that occurred earlier. The error line number is just where the parser finally gives up handling all errors.
Fixing Grammar Errors
There are many ways to narrow down and fix grammar issues.
-
Open the mentioned source file. Check out the mentioned line of code.
- For runaway strings and misplaced operators, you can usually find the culprit there.
- Read the line from left to right and imagine what each symbol does.
-
More commonly, you need to look at the line that precedes .
- In particular, the semicolon is missing; the semicolon is missing at the end of a previous line/statement. (At least from a style perspective)
- If the code block { } is not properly closed or nested, you may need to dig further into the source code. To do this, use appropriate code indentation.
-
Check out Syntax Coloring!
- Strings, variables and constants should all have different colors.
- operators -*/. should also be colored differently, otherwise they might be in the wrong context.
- If you see string shading extending too far or too short, then you have found an unescaped or missing closing " or ' string marker.
- If you see two Punctuation marks of the same color next to each other can also mean trouble. Usually, if it isn't, -- or parentheses following an operator, the operator is alone. Most of the time it is incorrect for two strings/identifiers to be directly adjacent to
-
spaces. Your friends follow any coding style. >
Temporarily split long lines -
You can freely add newlines
comment out problematic code. - If you can't determine the source of the problem, start commenting out (i.e. temporarily deleting) the code block.
As soon as you resolve the parsing error, you'll find the source of the problem.
Sometimes, you want to remove the complete function/method block temporarily. Curly brace mismatch and code indentation error)
- as If you can't solve the syntax problem, try
rewriting the commented out sections from scratch -
-
- to avoid some confusing syntax structures as a newbie. .
Ternary? : Conditional operator can reduce generation code, and does work. But it doesn't help readability in all cases. When not proficient, try to stick to ordinary if statements (if:/elseif:/endif; ) is often used for templates, but is arguably not as good as { code } blocks are easy to follow. > " or ' The string quotes do not match and there are unescaped quotes -
. Forget the operator, specifically the operator
for string . concatenation. The brackets are unbalanced ( ). Count them in the reported rows. Are their quantities equal? -
-
Don’t forget that solving one grammar problem may uncover the next.
- If you make one problem go away, but other problems appear in the code below, you're mostly on the right track.
- If a new syntax error appears in the same line after editing, your attempted changes may also fail. (But not always)
-
If you can't fix it, restore a backup of your previously working code.
- Adopt source code version control system. You can always see the difference between the corruption and the last working version. This might explain what the syntax problem is.
-
Invisible stray Unicode characters : In some cases you need to use a hex editor or a different editor for the source code /viewer. Some problems cannot be discovered by looking at the code.
- Try grep --color -P -n "[x80-xFF]" file.php as a first step to find non-ASCII symbols.
- In particular, BOMs, zero-width spaces, or non-breaking spaces and smart quotes often find their way into source code. -
-
Note the line break type saved in the file.
- PHP only supports the n line feed character and does not support the r carriage return character.
- This is occasionally an issue only for MacOS users (even on OS X which is used to misconfigurate the editor).
- When using single-line // or # comments, it usually only causes problems when newlines are ignored. Multiline /.../ comments rarely interfere with the parser when newlines are ignored.
-
If your grammar error is not transmitted over the network: There is a syntax error on your machine. But posting the exact same file online won't happen again. This can only mean one of two things:
- You are looking at the wrong file!
- Or your code contains invisible stray Unicode (see above). You can find out easily: just copy the code from the web form back into your text editor.
-
Check your PHP version. Not all syntax constructs may be available on every server.
- The command line interpreter's php -v
- called through the web server
Those are not necessarily the same. Especially when using frames, you need to make them match.
- Do not use PHP reserved keywords as identifiers for functions/methods, classes, or constants.
- Experimentation is your last resort.
If all else fails, you can always search the web for your error message. Syntax symbols are not easily searchable (Stack Overflow itself is indexed by SymbolHound). So it may take you several pages to find the relevant information.
Extended reading
- David Sklar's "PHP Debugging Basics"
- Jason McCreary's "Fixing PHP Errors"
- Mario Lurig's "PHP Errors—— 10 Common Mistakes》
- 《Common PHP Errors and Solutions》
- How to Troubleshoot and Fix Your WordPress Website
- A Designer’s Guide to PHP Error Messages - Smashing Magazine
White Dead Screen
If your website is just blank, the usual error is a syntax error. Enable their display using:
- error_reporting = E_ALL
- display_errors = 1
usually in your php.ini, or in the case of mod_php Via .htaccess and even for FastCGI settings via .user.ini.
It's too late to enable it in a broken script because PHP can't even interpret/run the first line. A quick fix is to make a wrapper script like test.php:
and then call the faulty code by accessing this wrapper script.
It is also helpful to enable PHP's error_log and look at the web server's error.log when the script crashes and receives an HTTP 500 response.
The above is the detailed content of PHP Syntax Errors: How to Troubleshoot and Resolve Them?. For more information, please follow other related articles on the PHP Chinese website!