How to Execute Bash Scripts from Go Effectively?
Executing Bash Scripts from Go
The Challenge
To execute a bash script from Go, you've attempted using the os/exec package but encountered challenges with inputting the script path or its content as arguments. This script sets variables and performs specific tasks.
The Solution
To successfully execute a bash script from Go, consider the following steps:
Prerequisites
- Ensure the bash script starts with #!/bin/sh or #!/bin/bash.
- Make the script executable by running chmod x
.
Using the os/exec Package
If you prefer using os/exec, modify your code as follows:
cmd := exec.Command("/bin/sh", mongoToCsvSH) out, err := cmd.Output()
Here, "/bin/sh" indicates the interpreter to execute the script, followed by the path to your bash script, mongoToCsvSH.
Alternative Approach
Instead of using os/exec, you can leverage the following code to execute the script:
import ( "io/ioutil" "os" ) func main() { content, err := ioutil.ReadFile("mongoToCsvSH.sh") if err != nil { log.Fatal(err) } err = os.WriteFile("run.sh", content, 0755) if err != nil { log.Fatal(err) } cmd := exec.Command("./run.sh") cmd.Run() }
This approach reads the bash script content, writes it to a temporary "run.sh" file with executable permission (chmod 0755), and then executes it.
The above is the detailed content of How to Execute Bash Scripts from Go Effectively?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


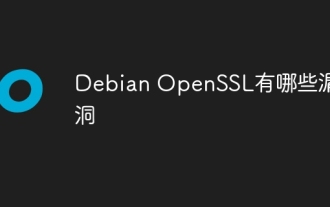
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
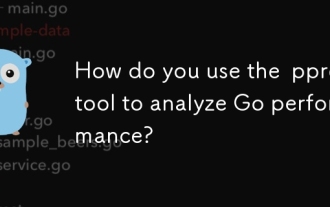
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
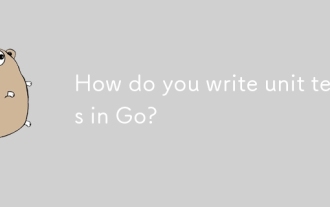
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
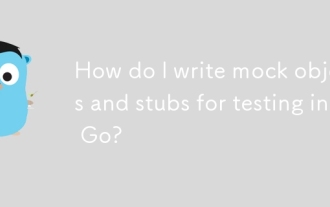
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
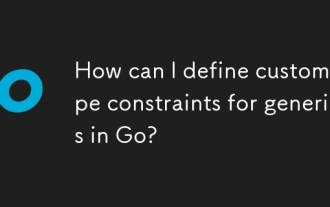
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
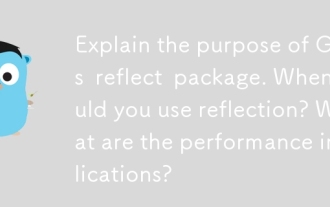
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
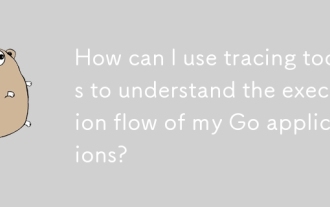
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
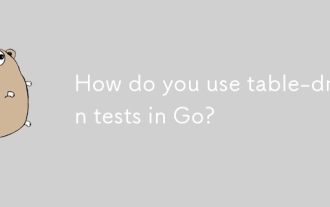
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
