


Why Does System.out.println and System.err.println Output Appear Out of Order?
Understanding Out-of-Order Printing with System.out.println and System.err.println
When using System.out.println and System.err.println, you might encounter an unexpected behavior where the output from these two methods appears out of order in the console.
Issue:
In the provided code:
public static void main(String[] args) { for (int i = 0; i < 5; i++) { System.out.println("out"); System.err.println("err"); } }
Output:
out out out out out err err err err err
Instead of alternating between "out" and "err," the output shows all "out" lines followed by all "err" lines.
Cause:
This behavior is due to the fact that System.out and System.err are different streams. When you write to these streams, the data is initially buffered. The buffered data is only written to the actual console output at specific times, such as when:
- The buffer is full.
- The program terminates.
- System.out.flush() or System.err.flush() is called.
By default, System.out and System.err are flushed differently. System.out is flushed more frequently than System.err. Therefore, any data written to System.out tends to appear before data written to System.err.
Solution:
To ensure that the output alternates between "out" and "err," you can call System.out.flush() and System.err.flush() inside the loop:
public static void main(String[] args) { for (int i = 0; i < 5; i++) { System.out.println("out"); System.err.println("err"); System.out.flush(); System.err.flush(); } }
With this modification, the output will alternate as expected:
out err out err out err out err out err
The above is the detailed content of Why Does System.out.println and System.err.println Output Appear Out of Order?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


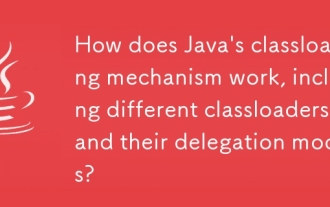
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
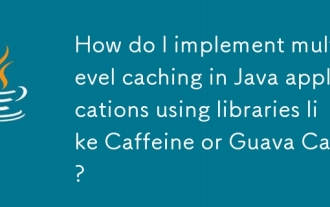
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
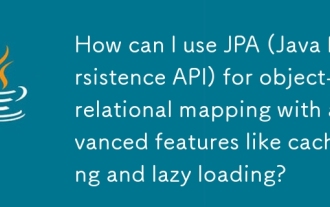
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
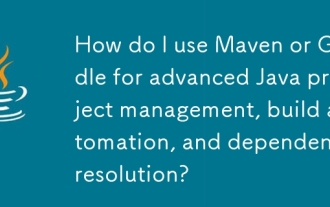
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
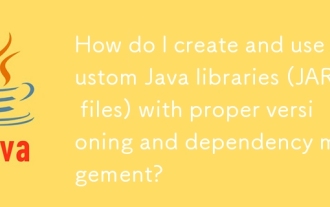
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
