


Solving the Empty Path Issue in Go Lambda Functions with API Gateway HTTP API
When deploying a Golang/Docker-based HTTP API to AWS Lambda, you may encounter an issue where the path property in the APIGatewayProxyRequest is empty. This occurs because the Lambda function is receiving events from API Gateway HTTP API (v2), which uses a different event structure compared to the REST API (v1).
The Problem
The APIGatewayProxyRequest is designed for the older REST API and will result in an empty path field when used with the HTTP API. The solution is to use the correct event structure: APIGatewayV2HTTPRequest.
The Solution
Switch to using the APIGatewayV2HTTPRequest struct, which includes the RawPath field to handle routing correctly.
The original code from their documentation (which doesn't work):
package main import ( "context" "github.com/aws/aws-lambda-go/events" "github.com/aws/aws-lambda-go/lambda" ) func handler(ctx context.Context, event events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) { response := events.APIGatewayProxyResponse{ StatusCode: 200, Body: "\"Hello from Lambda!\"", } return response, nil } func main() { lambda.Start(handler) }
Here’s an updated Lambda function example:
package main import ( "github.com/aws/aws-lambda-go/events" "github.com/aws/aws-lambda-go/lambda" ) func handler(request events.APIGatewayV2HTTPRequest) (*events.APIGatewayV2HTTPResponse, error) { if request.RawPath == "/health" { return &events.APIGatewayV2HTTPResponse{ StatusCode: 200, Body: "Health check passed", }, nil } return &events.APIGatewayV2HTTPResponse{ StatusCode: 404, Body: "Not Found", }, nil } func main() { lambda.Start(handler) }
Deploying Your Lambda
- Deploy the Lambda function to AWS.
- Create an HTTP API in API Gateway and integrate it with your Lambda function.
- Test the endpoint: Sending a request to /health should return a success message, while any other path returns a 404.
Conclusion
Switching to APIGatewayV2HTTPRequest resolves the issue of an empty path in your Lambda function when using API Gateway HTTP API (v2). Make sure to test your endpoint to ensure path routing works as expected.
Sourced from a github issue:
https://github.com/aws/aws-lambda-go/issues/60
The above is the detailed content of Solving the Empty Path Issue in Go Lambda Functions with API Gateway HTTP API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


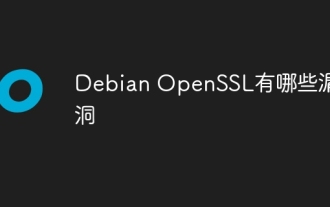
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
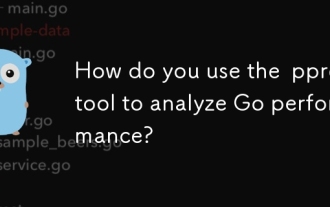
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
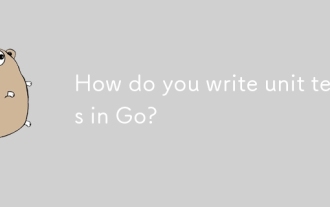
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
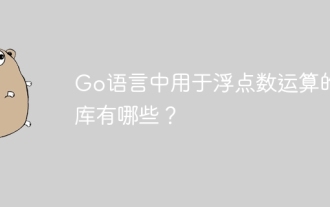
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
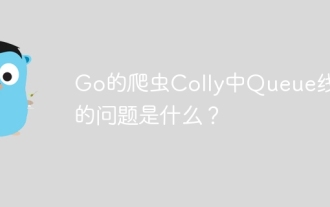
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
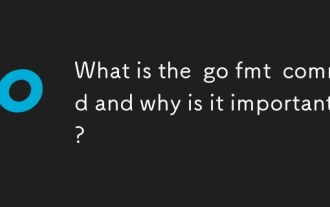
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
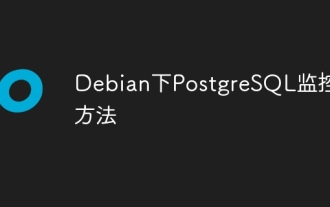
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
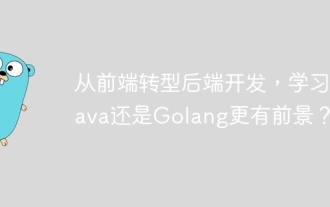
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
