How Can I Convert Objects to JSON Using jQuery?
Converting Objects to JSON with jQuery
In jQuery, serializing an object to JSON is a common task. To simplify this process, jQuery leverages the built-in JSON object supported by modern browsers. This object provides methods for both serialization and deserialization of JSON data.
To serialize an object into a JSON string, utilize the JSON.stringify() method:
var json_text = JSON.stringify(your_object, null, 2);
This method generates a string representing the object in JSON format, with optional indentation for enhanced readability.
For example, suppose you have an array of countries:
var countries = new Array(); countries[0] = 'ga'; countries[1] = 'cd'; ...
To convert this array into a JSON string suitable for passing to $.ajax(), apply JSON.stringify():
var json_text = JSON.stringify(countries);
This will produce a string like:
"['ga','cd']"
To deserialize a JSON string back into an object, utilize the JSON.parse() method:
var your_object = JSON.parse(json_text);
This method creates an object from the provided JSON string.
It is crucial to note that the JSON object is natively supported by most modern browsers. As a result, jQuery seamlessly integrates with this feature to provide simplified JSON handling.
The above is the detailed content of How Can I Convert Objects to JSON Using jQuery?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
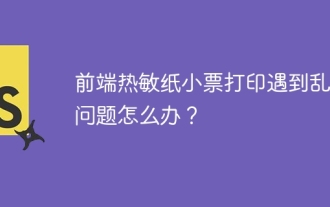
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
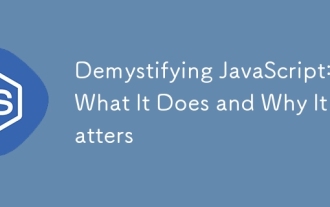
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
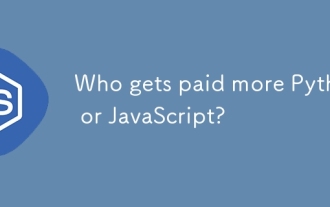
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
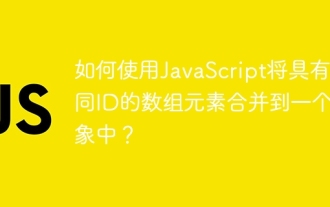
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
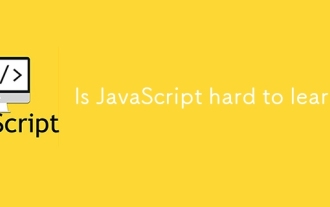
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
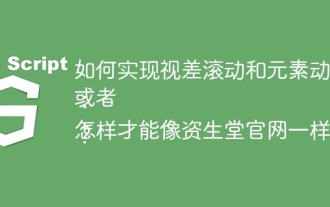
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
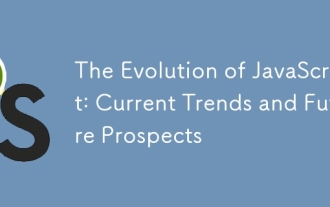
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
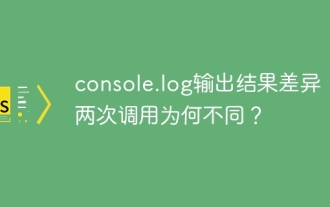
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
