What is the Use of the Tilde Token (~) in Go Generics?
Understanding the Tilde Token (~) in Go Generics
Go's introduction of the tilde token (~) has brought a new dimension to generic programming. This token allows developers to define types that share a common underlying type.
What is the Tilde Token?
In Go, the tilde token (~) signifies the set of all types with an underlying type T. For instance, ~T indicates all types whose underlying type is T.
Why is it Useful?
The tilde token provides a flexible way to define generic interfaces that can accept types with a specific underlying type. For example, an Ordered interface could be defined as:
type Ordered interface { Integer | Float | ~string }
This interface specifies that a type must be either an Integer, a Float, or a type whose underlying type is a string. This allows custom types with an underlying string type, such as MyString, to satisfy this interface.
Underlying Types
The concept of underlying types is crucial for understanding the tilde token. The underlying type of a type T is usually T itself, except:
- For predeclared numeric, boolean, or string types, the underlying type is the type itself.
- For composite types (structs, slices, etc.), the underlying type is the type to which the composite type refers in its type declaration.
Practical Examples
Using the tilde token, constraints can be created to limit the types that can satisfy a specific interface. For instance, a constraint called ExactSigned could be defined to only allow types that are exactly int, int8, int16, int32, or int64. Such a constraint would not allow custom types like MyInt8.
On the other hand, a constraint called constraints.Signed uses the ~ approximation element to allow types whose underlying type is int8. This allows custom types like MyInt8 to satisfy the constraint.
Syntactic Sugar
The tilde token can be used in unions, anonymous constraints, and even with syntactic sugar. Notably, you can use approximation elements with a single type to simplify writing constraints.
For example, the following anonymous constraints are equivalent:
func echoFixedSize[T interface { ~int8 | ~int32 | ~int64 }](t T) { /* ... */ } func echoFixedSizeSugar[T ~int8 | ~int32 | ~int64](t T) { /* ... */ } func echoFixedSizeSugarOne[T ~int8](t T) { /* ... */ }
Common Use Cases
Approximation elements are particularly useful for working with composite types that need to have methods. For instance, to allow a method to be declared for a ByteSeq type, its underlying type must be bound to the identifier:
type ByteSeq []byte func (b ByteSeq) DoSomething() {}
By specifying ~[]byte in a constraint, it becomes possible to instantiate ByteSeq as a valid type.
Limitations
It is important to note that the tilde token cannot be used with type parameters. For instance, the following code is invalid:
type AnyApprox[T any] interface { ~T }
The above is the detailed content of What is the Use of the Tilde Token (~) in Go Generics?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


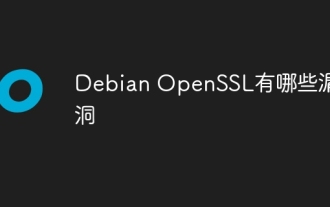
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
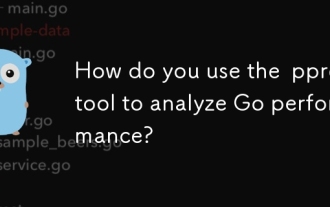
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
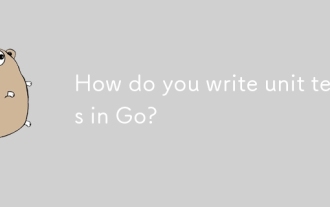
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
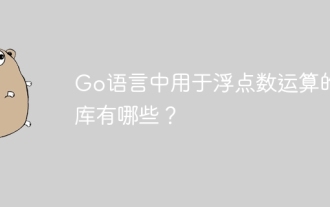
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
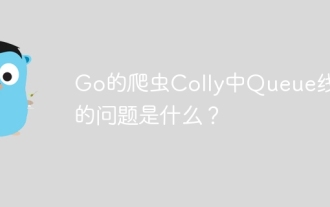
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
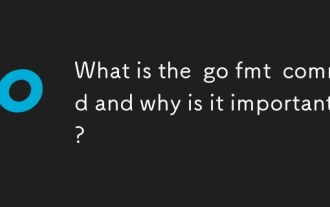
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
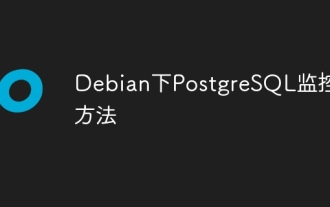
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
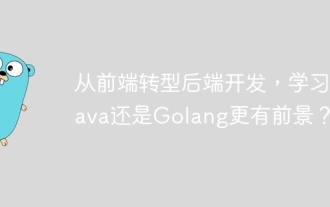
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
