


How Can I Efficiently Transpose Multidimensional Arrays in PHP?
Transposing Multidimensional Arrays in PHP: Flipping and Generalizing
Transposing a multidimensional array is an operation that flips rows and columns, effectively rotating the array 90 degrees. To address this common programming task, let's explore a PHP function that accomplishes this for both two-dimensional and higher-dimensional arrays.
Transposing Two-Dimensional Arrays
For a two-dimensional array, such as the example provided in the question, transposing it is a straightforward process. The following function, named transpose, achieves this:
function transpose($array) { array_unshift($array, null); return call_user_func_array('array_map', $array); }
This function works by adding a null element as the first index of the array and then using array_map to iterate over each row and create a new array of the respective columns.
For instance, to transpose the $foo array given in the question:
$bar = transpose($foo); var_dump($bar[2]);
This will output the desired result:
array(3) { ["a"]=> string(2) "a2" ["b"]=> string(2) "b2" ["c"]=> string(2) "c2" }
Generalizing to Higher-Dimensional Arrays
The transpose function can be generalized to handle arrays of any dimension using recursion. Here's how:
function multi_transpose($array) { if (!is_array($array)) { return array($array); } $transposed = array(); foreach ($array as $key => $value) { $transposed[] = multi_transpose($value); } return call_user_func_array('array_map', $transposed); }
This function takes an array as input and recursively calls itself on the array's values. The base case is when the value is no longer an array, in which case it returns a one-element array containing the value. For arrays, it recursively transposes each value and uses array_map to combine them into the final transposed array.
Using the multi_transpose function is similar to the two-dimensional transpose function:
$multi_dimensional_array = ...; $transposed = multi_transpose($multi_dimensional_array);
This function can handle arbitrarily nested arrays and will transpose them by flipping their dimensions 90 degrees.
The above is the detailed content of How Can I Efficiently Transpose Multidimensional Arrays in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


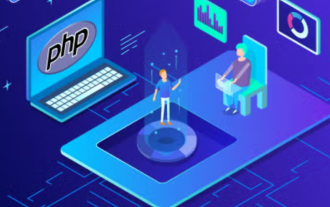
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
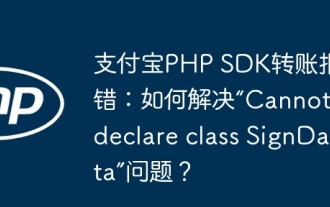
Alipay PHP...
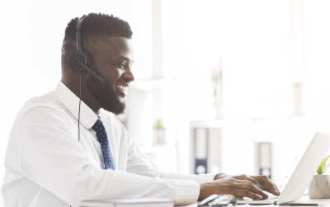
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
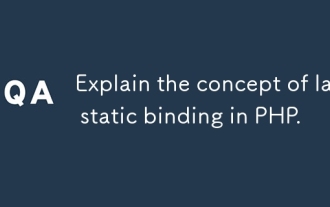
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
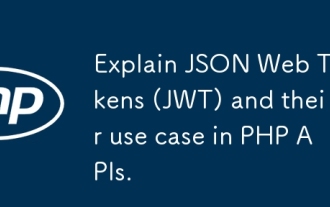
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
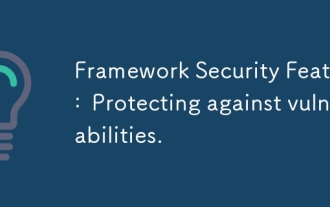
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
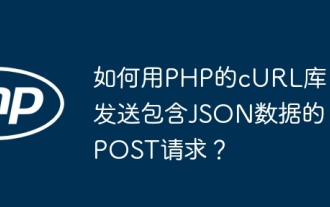
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
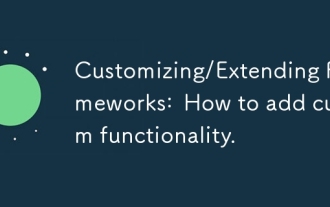
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
