How Can I Use Capturing Groups in Go Regular Expressions?
Capturing Groups in Go Regular Expressions
When porting code from Ruby to Go, developers often encounter compatibility issues with regular expressions. Ruby supports capturing groups within regexes, a feature not natively available in Go's RE2 library.
Rewriting Expressions for Go
To emulate capturing group functionality, the following steps can be taken:
1. Use Named Capture Groups:
Instead of using angle brackets, add "P" between the opening parenthesis and the group name, e.g., (?P
2. Cross-Reference Group Names:
Utilize the regexp.SubexpNames() function to obtain a list of capture group names.
3. Access Captured Data:
Use the appropriate group name as an argument to functions like FindStringSubmatch to retrieve the captured values.
Example:
package main import ( "fmt" "regexp" ) func main() { r := regexp.MustCompile(`(?P<Year>\d{4})-(?P<Month>\d{2})-(?P<Day>\d{2})`) s := `2015-05-27` fmt.Printf("Matched Substrings: %#v\n", r.FindStringSubmatch(s)) fmt.Printf("Group Names: %#v\n", r.SubexpNames()) }
Output:
Matched Substrings: [2015 05 27] Group Names: [ Year Month Day ]
The above is the detailed content of How Can I Use Capturing Groups in Go Regular Expressions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


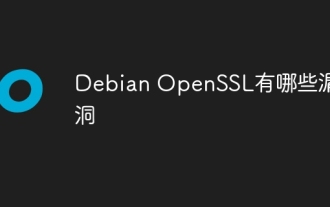
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
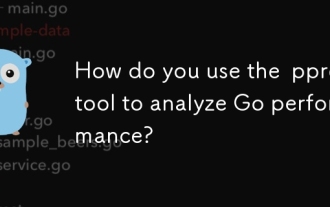
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
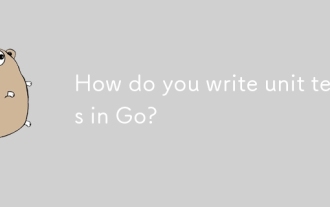
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
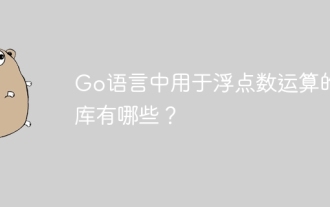
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
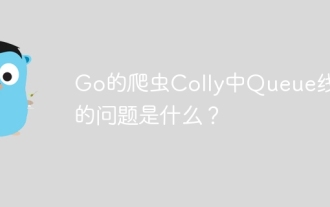
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
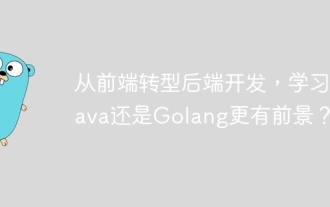
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
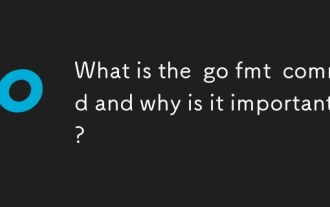
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
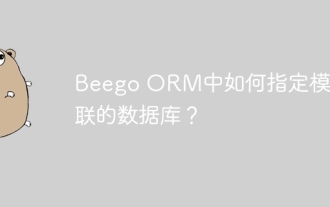
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
