Building a Real-Time Chat Application with WebSockets in React
Real-time communication has become an integral feature of modern web applications, especially in chat applications. WebSockets provide a powerful way to enable real-time, bidirectional communication between a client and a server. In this guide, we’ll walk through the process of building a real-time chat application using WebSockets and React.
Prerequisites
Before diving in, ensure you have the following:
- Basic understanding of React.
- Node.js installed on your machine.
- A package manager like npm or yarn.
- Familiarity with WebSocket concepts.
Step 1: Setting Up the Backend
We need a WebSocket server to handle real-time communication. We'll use Node.js with the ws package.
- Initialize a Node.js project:
mkdir chat-backend cd chat-backend npm init -y
- Install the ws package:
npm install ws
- Create a WebSocket server:
// server.js const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', (ws) => { console.log('Client connected'); ws.on('message', (message) => { console.log(`Received: ${message}`); // Broadcast the message to all clients wss.clients.forEach((client) => { if (client.readyState === WebSocket.OPEN) { client.send(message); } }); }); ws.on('close', () => { console.log('Client disconnected'); }); }); console.log('WebSocket server running on ws://localhost:8080');
- Run the server:
node server.js
Step 2: Setting Up the React Frontend
- Create a new React app:
npx create-react-app chat-frontend cd chat-frontend
- Install dependencies for WebSocket: No additional dependencies are required as the browser's native WebSocket API will be used.
Step 3: Building the Chat Interface
- Create a Chat component:
// src/components/Chat.js import React, { useState, useEffect, useRef } from 'react'; const Chat = () => { const [messages, setMessages] = useState([]); const [input, setInput] = useState(''); const ws = useRef(null); useEffect(() => { ws.current = new WebSocket('ws://localhost:8080'); ws.current.onmessage = (event) => { setMessages((prev) => [...prev, event.data]); }; return () => { ws.current.close(); }; }, []); const sendMessage = () => { if (input.trim()) { ws.current.send(input); setInput(''); } }; return ( <div> <ol> <li>Use the Chat component in your App.js: </li> </ol> <pre class="brush:php;toolbar:false">import React from 'react'; import Chat from './components/Chat'; function App() { return <Chat />; } export default App;
- Start the React app:
npm start
Step 4: Testing the Application
- Open your React app in multiple tabs or devices.
- Start typing messages in one tab, and observe them appear in all connected clients in real-time!
Additional Enhancements
To make the app production-ready, consider:
- Adding user authentication for personalized messages.
- Integrating a database to store chat history.
- Deploying the WebSocket server and React app to platforms like Vercel, Heroku, or AWS.
Conclusion
By leveraging WebSockets, we’ve built a lightweight, real-time chat application using React. This project demonstrates the power of WebSockets for dynamic communication, which is useful in various applications like messaging platforms, gaming, and live notifications. Dive deeper into WebSocket protocols to enhance your application further!
Happy coding! ?
The above is the detailed content of Building a Real-Time Chat Application with WebSockets in React. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










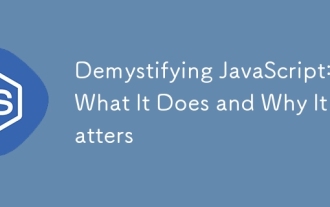
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
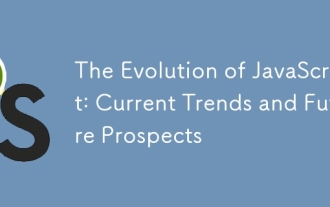
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
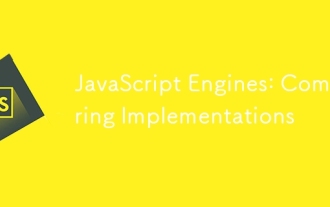
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
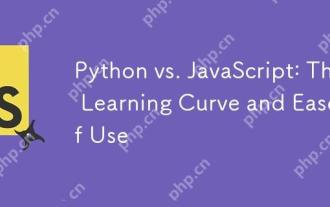
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
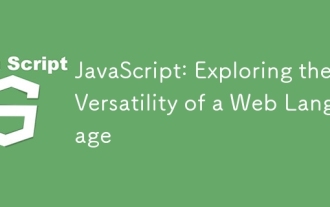
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
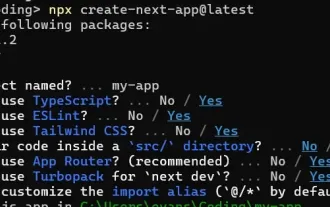
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
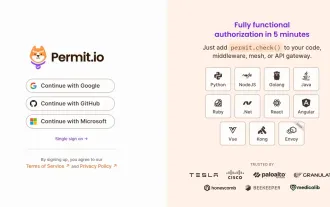
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
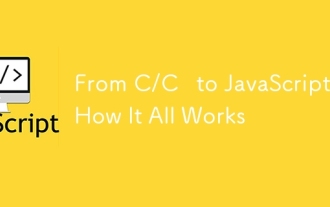
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
