How Can I Define a Recursive Type Constraint in Go 2 Generics?
How to Define a Recursive Type Constraint Using a Custom Interface
In Go2 generics, you can use an interface to constrain a generic type, ensuring it implements a specific method. However, enforcing the implementation of a method with an argument of the generic type was not allowed in the current draft.
Introducing a Solution:
The new draft allows for defining the Lesser interface and IsLess function as:
type Lesser[T any] interface { Less(T) bool } func IsLess[T Lesser[T]](x, y T) bool { return x.Less(y) }
This solution enforces that T must be of a type that implements the Lesser interface, which requires a Less method taking an argument of type T. By defining the constraint T Lesser[T], we create a recursive type constraint.
Practical Example:
type Apple int func (a Apple) Less(other Apple) bool { return a < other } type Orange int func (o Orange) Less(other Orange) bool { return o < other } func main() { fmt.Println(IsLess(Apple(10), Apple(20))) // true fmt.Println(IsLess(Orange(30), Orange(15))) // false }
In this example, the custom types Apple and Orange both fulfill the Lesser requirement and can be passed to IsLess. However, passing an int or mixing types (e.g., Apple and Orange) will result in compilation errors due to type constraints.
Conclusion:
This solution allows for recursive type constraints using a defined interface in the new Go2 generics draft, allowing you to enforce complex relationships between generic types and their methods.
The above is the detailed content of How Can I Define a Recursive Type Constraint in Go 2 Generics?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


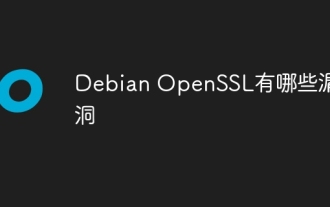
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
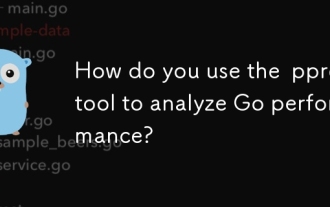
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
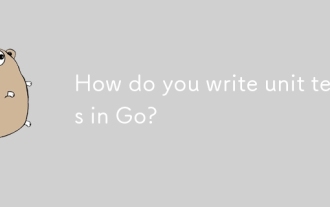
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
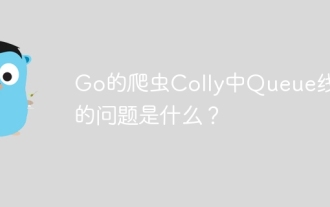
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
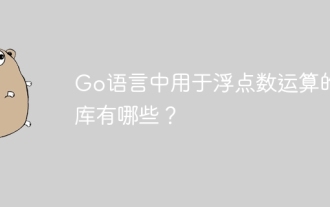
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
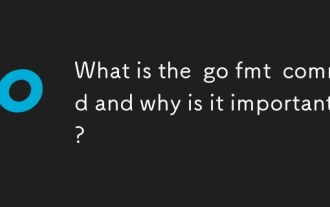
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
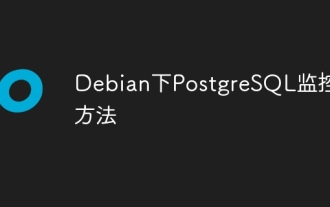
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
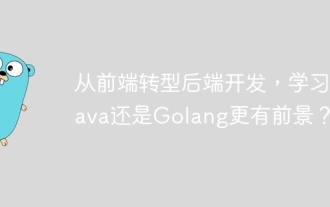
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
