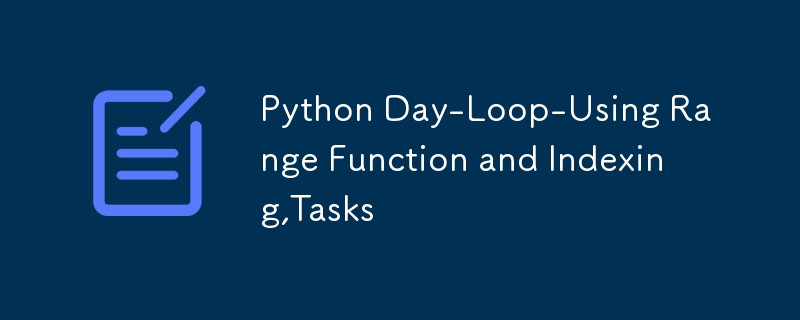
Fibonnaci sequence:
1) Using 3 variables:
1 2 3 4 5 6 | f, s = -1, 1
t = 0
while t<=13:
t= f + s
print (t, end = ' ' )
f,s = s, t
|
Copy after login
Output:
2) Using 2 variables:
1 2 3 4 | f, s = -1, 1
while f+s<=13:
print (f + s, end = ' ' )
f,s = s, f + s
|
Copy after login
Output:
Range function:
range() function is used to generate a sequence of numbers. It is commonly used in loops for iterating a specific number of times.
Syntax:
range(start, stop, step)
-->start (optional): The starting number of the sequence. Defaults to 0 if not specified.
-->stop (required): The number where the sequence ends (exclusive, i.e., not included in the output).
-->step (optional): The increment or decrement value. Defaults to 1 if not specified.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 | print ( "First Output" )
for no in range(10):
print (no, end = ' ' )
print ( "\nSecond Output" )
for no in range(1,10):
print (no, end = ' ' )
print ( "\nThird Output" )
for no in range(5,10):
print (no, end = ' ' )
print ( "\nFourth Output" )
for no in range(1,10,2):
print (no, end = ' ' )
print ( "\nFifth Output" )
for no in range(3,15,3):
print (no, end = ' ' )
print ( "\nSixth Output" )
for no in range(10,1):
print (no, end = ' ' )
print ( "\nSeventh Output" )
for no in range(10,1,-1):
print (no, end = ' ' )
print ( "\nEighth Output" )
for no in range(20,3,-1):
print (no, end = ' ' )
print ( "\nNineth Output" )
for no in range(20,2,-2):
print (no, end = ' ' )
|
Copy after login
Output:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | First Output
0 1 2 3 4 5 6 7 8 9
Second Output
1 2 3 4 5 6 7 8 9
Third Output
5 6 7 8 9
Fourth Output
1 3 5 7 9
Fifth Output
3 6 9 12
Sixth Output
Seventh Output
10 9 8 7 6 5 4 3 2
Eighth Output
20 19 18 17 16 15 14 13 12 11 10 9 8 7 6 5 4
Nineth Output
20 18 16 14 12 10 8 6 4
|
Copy after login
Explanation for sixth output:
The range() function requires a step parameter to generate a sequence in reverse. When the step is not specified, it takes 1 as default, which means the sequence will try to increment from 10 to 1, but since 10 is greater than 1, no numbers are generated.
Negative indexing:
Normally indexing starts from 0 but it can also be started from -1 which means negative indexing(starts from -1).
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | name = 'ABCDEFGHI'
for letter in name[0:5]:
print (letter, end = ' ' )
print ()
for letter in name[0:6:2]:
print (letter, end = ' ' )
print ()
for letter in name[8:0:-1]:
print (letter, end = ' ' )
print ()
for letter in name[8:2:-1]:
print (letter, end = ' ' )
print ()
for letter in name[8:-1:-1]:
print (letter, end = ' ' )
print ()
for letter in name[8:3:-2]:
print (letter, end = ' ' )
print ()
for letter in name[8::-1]:
print (letter, end = ' ' )
print ()
for letter in name[::]:
print (letter, end = ' ' )
print ()
for letter in name[6::]:
print (letter, end = ' ' )
print ()
for letter in name[2::2]:
print (letter, end = ' ' )
|
Copy after login
Output:
1 2 3 4 5 6 7 8 9 10 | A B C D E
A C E
I H G F E D C B
I H G F E D
I G E
I H G F E D C B A
A B C D E F G H I
G H I
C E G I
|
Copy after login
Explanation:Fifth output()
name[8:-1:-1]
In this indexing start is 8 which in above example is the last value,end -1 also indicates last value so output has returned nothing.
Find palindrome or not for given string:
1 2 3 4 5 | name = input( "Enter word: " )
if name[::] == name[::-1]:
print ( "Palindrome" )
else :
print ( "Not Palindrome" )
|
Copy after login
Output:
1 2 | Enter word: amma
Palindrome
|
Copy after login
Pattern formation:
Example:1
1 2 | for num in range(1,6):
print ( "* " * num)
|
Copy after login
Output:
1 2 3 4 5 | *
* *
* * *
* * * *
* * * * *
|
Copy after login
Example:2
1 2 | for num in range(5,0,-1):
print ( "* " * num)
|
Copy after login
Output:
1 2 3 4 5 | * * * * *
* * * *
* * *
* *
*
|
Copy after login
Note: * works between 2 strings but will not work between 2 strings.(for eg- a*2-->aa,a 2-->a2)
Example:3
1 2 3 4 5 | digit = "1"
for num in range(5,0,-1):
print (digit * num)
digit = str(int(digit)+1)
print ()
|
Copy after login
Output:
Tasks:
word = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
1)ABCDEFGHI
2)XYZ
3)ZYXWV
4)ACEGI
5)IGECA
6)ZXVTRPNLJHFDB
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | word = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
print ( "First Output" )
for letter in word[0:9]:
print (letter , end = " " )
print ( "\nSecond Output" )
for letter in word[23::]:
print (letter , end = " " )
print ( "\nThird Output" )
for letter in word[-1:-6:-1]:
print (letter , end = " " )
print ( "\nFouth Output" )
for letter in word[0:9:2]:
print (letter , end = " " )
print ( "\nFifth Output" )
for letter in word[8::-2]:
print (letter , end = " " )
print ( "\nSixth Output" )
for letter in word[-1::-2]:
print (letter , end = " " )
|
Copy after login
Output:
1 2 3 4 5 6 7 8 9 10 11 12 | First Output
A B C D E F G H I
Second Output
X Y Z
Third Output
Z Y X W V
Fouth Output
A C E G I
Fifth Output
I G E C A
Sixth Output
Z X V T R P N L J H F D B
|
Copy after login
The above is the detailed content of Python Day-Loop-Using Range Function and Indexing,Tasks. For more information, please follow other related articles on the PHP Chinese website!