


How Can I Achieve C's getchar() Functionality, Including Tab Handling, in Go?
A Go Function Analogous to C's getchar
In C programming, the getchar() function is commonly used for character input handling. This function waits for a single keystroke from the user and returns its ASCII value. For advanced terminal applications, this functionality becomes indispensable for features such as autocompletion. However, in Go, there is no direct equivalent to getchar() that caters to tab press handling.
Go's Approach to Character Input
To achieve similar functionality in Go, we can employ the bufio.NewReader() and os.Stdin packages. Here's an example:
package main import ( "bufio" "fmt" "os" ) func main() { reader := bufio.NewReader(os.Stdin) input, _ := reader.ReadString('\n') fmt.Printf("Input Char Is : %v", string([]byte(input)[0])) }
This code reads a line of input from the user and prints the ASCII value of the first character. To detect tab presses specifically, you can check if the first element of the input is a tab character (U 0009).
Limitations of getchar() for Tab Handling
It's important to note that getchar() is not ideal for tab handling because it requires the user to press enter after inputting a character. This behavior doesn't align well with the expected functionality for tab autocompletion.
Alternative Approaches
To implement autocompletion effectively, you'll need to consider other options:
- Use ncurses Binding: There are Go bindings available for ncurses, a popular terminal handling library, which provides functions like getch() for single-keystroke input handling.
- Create a Custom Solution: You can create your own Go implementation to handle raw input and keystrokes, which would give you full control over the behavior.
- Use os.Exec: You can execute external commands using os.Exec, such as stty or jLine, which provide similar functionality to getchar().
Conclusion
While Go does not have an exact analog to C's getchar(), you can leverage various techniques to achieve similar functionality, depending on your specific requirements. Whether you choose to use ncurses bindings, create a custom solution, or utilize os.Exec, the goal is to implement effective keystroke and tab handling for your Go applications.
The above is the detailed content of How Can I Achieve C's getchar() Functionality, Including Tab Handling, in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


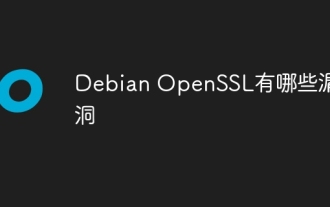
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
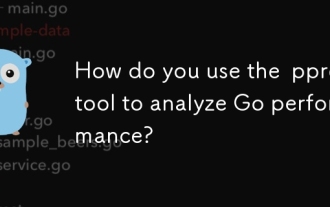
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
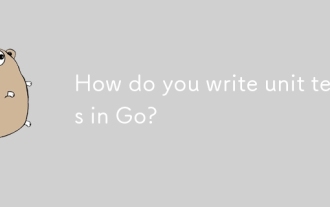
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
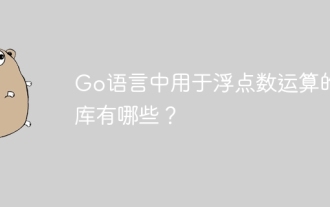
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
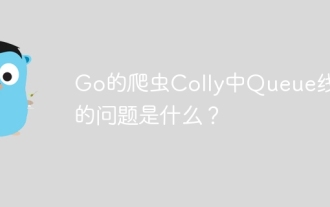
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
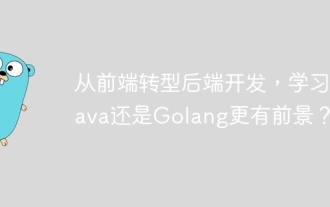
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
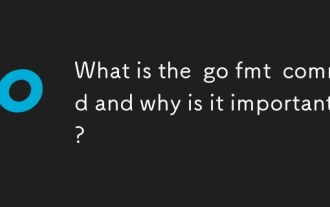
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
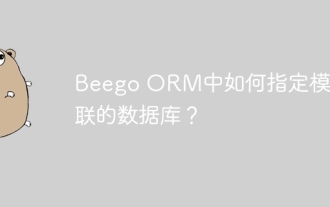
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
