


Why Can't I Access Class Variables Directly in Python 3 List Comprehensions Within a Class Definition?
Accessing Class Variables from a List Comprehension in the Class Definition
In Python 3, accessing class variables from a list comprehension within the class definition is not allowed due to scoping limitations. The class scope is not considered a valid lookup scope for variables used in the list comprehension.
Error Example:
class Foo: x = 5 y = [x for i in range(1)]
This code would raise a NameError: name 'x' is not defined in Python 3.
Cause: Scoping Restrictions
Python follows strict scoping rules, and the class scope is separate from the scope of functions, loops, and comprehensions. Comprensions always execute within their own scope, which includes any variables declared within the comprehension itself.
In Python 2, this was not an issue because list comprehensions were implemented using a shortcut that allowed access to the enclosing class scope. However, this behavior was considered an inconsistency and was changed in Python 3 to enforce proper scoping.
Exception: Outermost Iterable
While the innermost iterable of a list comprehension can't access class variables, the outermost iterable expression can. This is because the outermost iterable is evaluated in the surrounding scope:
class Foo: x = 5 y = [i for i in range(x)] # This works fine
Workarounds:
Explicit Function:
Create a function within the class that has access to class variables and uses a list comprehension:
class Foo: x = 5 def get_y(self): return self.x, [x for i in range(self.x)]
Instance Variable:
Initialize an instance variable in the constructor using a list comprehension:
class Foo: def __init__(self): self.y = [self.x for i in range(1)]
Global Variable:
Declare the variable outside the class and use it in the list comprehension:
x = 5 class Foo: y = [x for i in range(1)]
The above is the detailed content of Why Can't I Access Class Variables Directly in Python 3 List Comprehensions Within a Class Definition?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
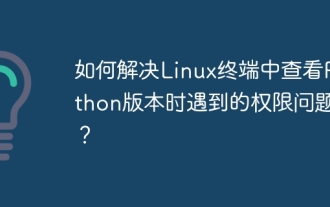
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
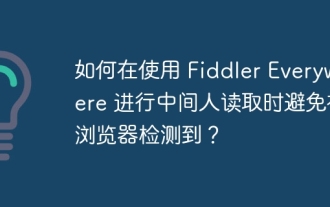
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
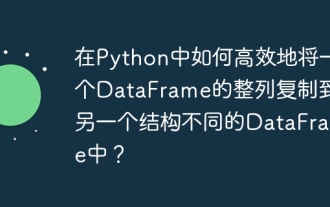
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
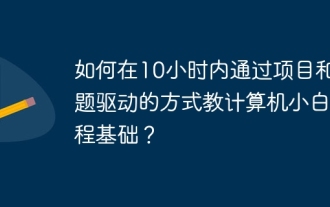
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
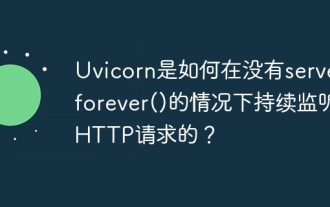
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
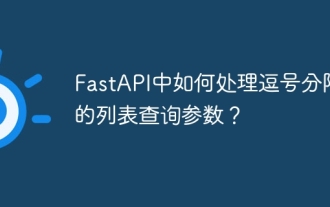
Fastapi ...
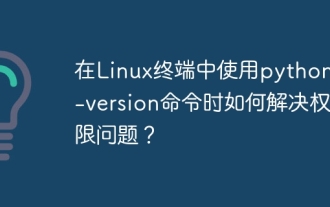
Using python in Linux terminal...
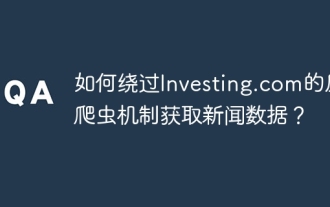
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
