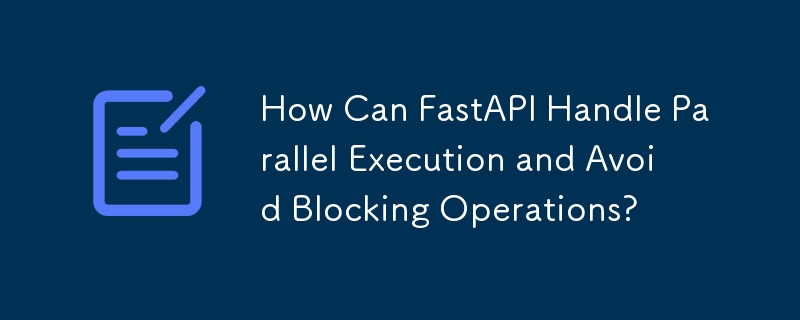
FastAPI and Parallel Execution
Asynchronous Programming in FastAPI
By default, FastAPI employs asynchronous programming patterns, allowing it to handle multiple requests concurrently. Specifically, it uses coroutines and the event loop to execute requests in an efficient manner.
Def vs. Async Def Functions
Functions defined as def (synchronous) are processed in a separate thread within an external threadpool. Functions defined as async def (asynchronous) are directly executed in the event loop.
Running Blocking Code
Problem: When a blocking operation is run within an async def endpoint, it can block the event loop and serialize request processing.
Solution:
-
Define Endpoints with def: If the endpoint does not require asynchronous operations, declare it with a normal def to avoid blocking.
-
Use run_in_threadpool(): For blocking operations within async def endpoints, the FastAPI run_in_threadpool() function allows you to run the task in a separate thread within the threadpool to prevent event loop blockage.
-
Use asyncio.loop.run_in_executor(): This function provides an alternative to run_in_threadpool() for executing blocking tasks asynchronously.
-
Use a separate process (ProcessPoolExecutor): For CPU-intensive computations, it's recommended to run the task in a separate process to maximize parallelization.
Avoiding Thread Pool Exhaustion
- Use the httpx library along with asyncio.gather() for executing multiple asynchronous HTTP requests in parallel.
- Employ multiple FastAPI workers (uvicorn --workers) to distribute requests across multiple processes, each with its own threadpool.
- Consider using external job queuing systems like Celery for heavy background computations.
Additional Notes:
-
Browser Caching: Open Incognito tabs or use a different browser session to avoid browser caching effects on API calls.
-
Asynchronous I/O: Asynchronous HTTP clients like httpx and aiohttp provide better performance than synchronous clients like requests.
The above is the detailed content of How Can FastAPI Handle Parallel Execution and Avoid Blocking Operations?. For more information, please follow other related articles on the PHP Chinese website!