


How Can I Correctly Read UTF-16 Text Files in Go, Handling Both BOM and Non-BOM Encodings?
Reading UTF-16 Text File as a String in Go
When reading UTF-16 text files in Go, you may encounter issues with bytes being interpreted as ASCII. This arises because the standard bufio.NewReader function doesn't handle unicode correctly.
Solution
UTF-16 with BOM
The latest version of "golang.org/x/text/encoding/unicode" introduces unicode.BOMOverride, which automatically detects and interprets the BOM to decode UTF-16 correctly. Here's an example using ReadFileUTF16():
func ReadFileUTF16(filename string) ([]byte, error) { raw, err := ioutil.ReadFile(filename) if err != nil { return nil, err } win16be := unicode.UTF16(unicode.BigEndian, unicode.IgnoreBOM) utf16bom := unicode.BOMOverride(win16be.NewDecoder()) unicodeReader := transform.NewReader(bytes.NewReader(raw), utf16bom) decoded, err := ioutil.ReadAll(unicodeReader) return decoded, err }
This function will decode UTF-16 files with a BOM.
UTF-16 without BOM
If your file does not contain a BOM, you can use the following code:
func ReadFileUTF16WithoutBOM(filename string) ([]byte, error) { f, err := os.Open(filename) if err != nil { return nil, err } r := bufio.NewReader(f) // Read past the BOM, if any. var b, e = r.Peek(2) if (b[0] == 0xFF && b[1] == 0xFE) || (b[0] == 0xFE && b[1] == 0xFF) { r.Discard(2) } // Read the rest of the file. decoded, err := ioutil.ReadAll(r) return decoded, err }
This function will skip any BOM and read the file as UTF-16.
Conclusion
By using ReadFileUTF16() or ReadFileUTF16WithoutBOM(), you can handle both BOM and non-BOM UTF-16 text files in Go, ensuring accurate decoding and representation of your data.
The above is the detailed content of How Can I Correctly Read UTF-16 Text Files in Go, Handling Both BOM and Non-BOM Encodings?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


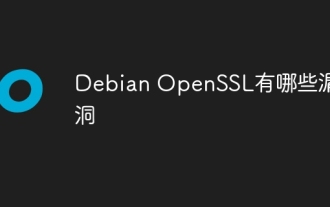
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
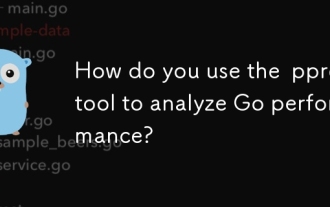
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
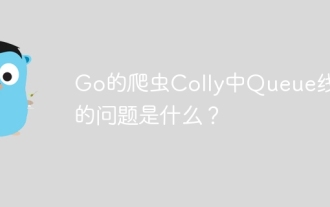
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
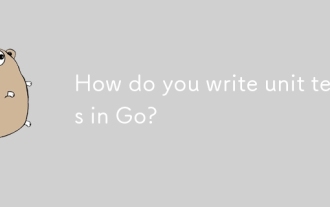
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
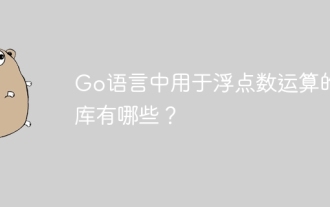
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
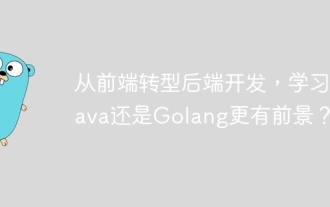
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
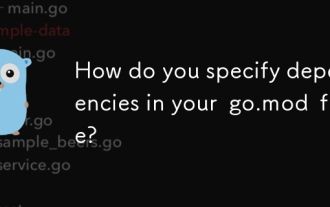
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
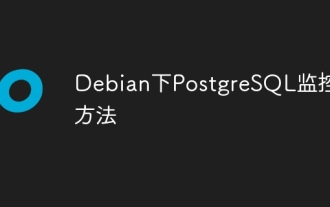
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
