


How Does Python's `getattr()` Function Work, and When Should I Use It?
What is and How to Use getattr() in Python?
Introduction:
getattr() is a built-in Python function that enables you to dynamically access an attribute of an object by passing its name as a string. This provides flexibility and versatility when working with objects and dynamically accessing their properties.
Description:
getattr(object, name, default=None) returns the attribute of the 'object' that is named by 'name'. If the attribute does not exist, it raises an AttributeError exception. However, you can specify a 'default' value to be returned instead.
Usage Scenarios:
When you need to access an attribute of an object but its name is stored in a variable or determined at runtime, getattr() becomes useful. Consider the following code snippet:
class Person: name = "John" age = 25 attr_name = "age" person = Person() # Access attribute dynamically using getattr() age = getattr(person, attr_name)
In this example, the 'age' attribute is accessed using getattr(). The first argument is the object (person), the second is the attribute name (attr_name), and the third argument (default=None) is not used since the attribute exists.
Iteration with getattr() and dir():
You can also use getattr() in conjunction with the dir() function to iterate over all attributes of an object and obtain their values. The following code demonstrates this:
for attr_name in dir(person): attr_value = getattr(person, attr_name) # Do something with attr_name and attr_value
Practical Applications:
- Dynamically invoking methods whose names are determined at runtime
- Getting attribute references to perform introspection and metadata analysis
- Iterating over all attributes of an object
- Accessing and modifying attributes whose names are stored in variables or obtained from external sources
Conclusion:
getattr() is a powerful tool for dynamically accessing and manipulating attributes of objects in Python. It offers flexibility and allows for complex attribute retrieval scenarios, making it an essential technique for advanced Python development.
The above is the detailed content of How Does Python's `getattr()` Function Work, and When Should I Use It?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
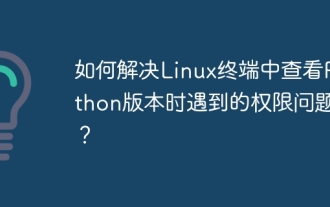
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
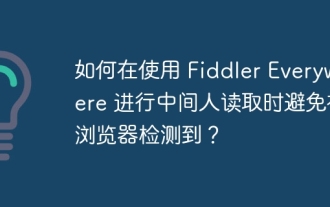
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
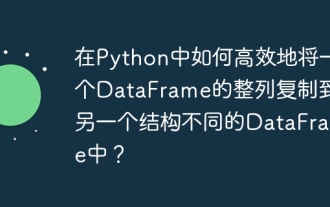
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
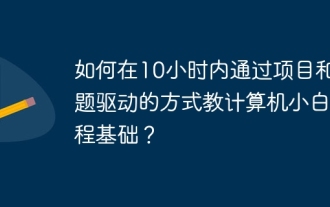
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
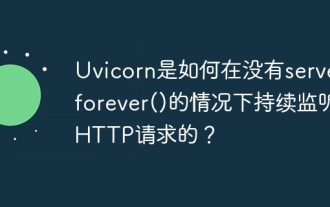
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
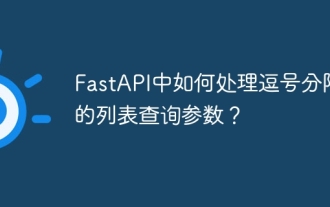
Fastapi ...
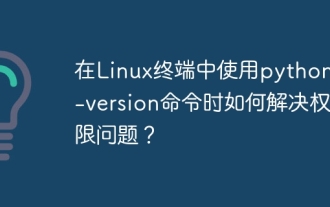
Using python in Linux terminal...
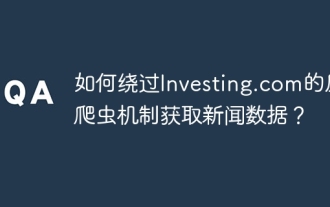
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
