How to Calculate a File's MD5 Checksum in Java?
Finding a File's MD5 Checksum in Java: A Detailed Guide
It's understandable to be perplexed about the absence of information regarding file MD5 checksums in Java. This article aims to fill that gap and provide a comprehensive guide on how to achieve this in Java.
The Essence of MD5 Checksums
MD5 (Message Digest 5) is a cryptographic hash function that produces a 128-bit message digest from a file. It's widely used to verify file integrity and detect any alterations or corruptions.
Computing MD5 Checksums in Java
Java offers a convenient way to compute MD5 checksums using the MessageDigest and DigestInputStream classes. Here's a breakdown of the steps involved:
- Creating a Message Digest: Create a MessageDigest object for the "MD5" algorithm, which will generate the message digest.
- Creating a Digest Input Stream: Wrap the file's input stream in a DigestInputStream object. This stream delegates read operations to the underlying input stream while also updating the message digest.
- Processing the File: Read bytes from the DigestInputStream as usual. As you do this, the message digest is computed in the background.
- Retrieving the Digest: After processing, call the digest() method of the MessageDigest object to obtain the 128-bit message digest.
Example Code
The following code snippet demonstrates how to compute the MD5 checksum of a file:
import java.io.File; import java.io.FileInputStream; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Paths; import java.security.DigestInputStream; import java.security.MessageDigest; public class ComputeMD5Checksum { public static void main(String[] args) throws IOException { MessageDigest md = MessageDigest.getInstance("MD5"); try (InputStream is = new FileInputStream("file.txt"); DigestInputStream dis = new DigestInputStream(is, md)) { // Read the file using the DigestInputStream } byte[] digest = md.digest(); // Process and display the digest as needed } }
Conclusion
Leveraging Java's MessageDigest and DigestInputStream classes allows for straightforward computation of MD5 checksums from files. By employing this technique, developers can ensure file integrity, detect alterations, and enhance data security.
The above is the detailed content of How to Calculate a File's MD5 Checksum in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
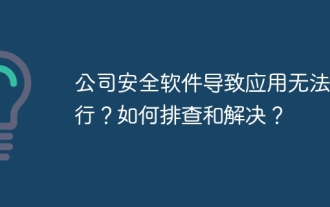
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
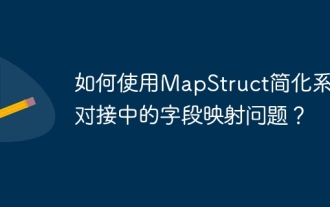
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
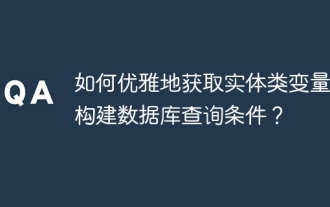
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
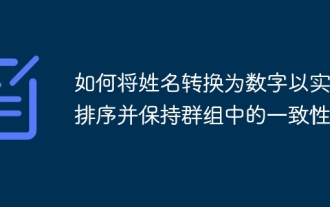
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
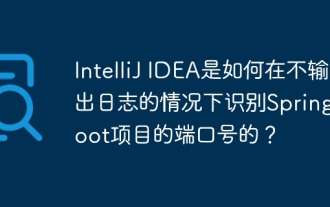
Start Spring using IntelliJIDEAUltimate version...
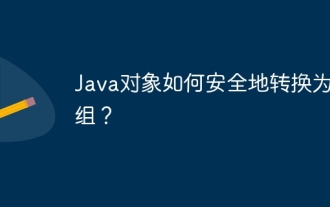
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
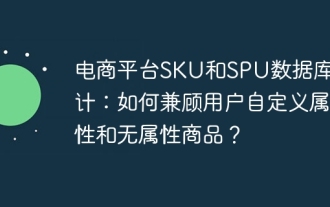
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
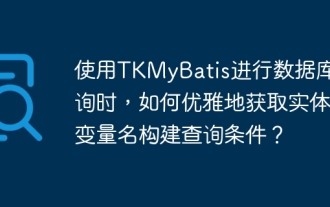
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
