


Why Does Modifying a Python List During Iteration Lead to Unexpected Results?
Iterative List Alteration in Python: Understanding the Consequences
In Python, modifying a list while iterating over it can lead to unexpected results. Consider the following code:
numbers = list(range(1, 50)) for i in numbers: if i < 20: numbers.remove(i) print(numbers)
Instead of removing numbers below 20, as intended, this code prints a list where all numbers below 20 are missing, along with 20 itself.
The Problem: Iterator Position Shift
The issue stems from the fact that removing an item from a list shifts the position of subsequent items. As the loop progresses, the items being checked are no longer the expected ones, as the removed elements create "holes" in the list.
For instance, during the initial iteration, 1 is removed, but the next iteration does not check 2; instead, it checks 3 because the list has been shortened. This behavior continues, resulting in the incorrect output.
Proper List Alteration Techniques
To avoid this problem, use alternative methods for altering lists during iteration, such as:
- List Comprehension:
numbers = [n for n in numbers if n >= 20]
- In-place Alteration:
numbers[:] = (n for n in numbers if n >= 20)
- Enumeration:
for i, n in enumerate(numbers): if n < 20: numbers[i] = None numbers = [n for n in numbers if n is not None]
These techniques do not modify the list's length while iterating, ensuring correct item processing and the desired output.
The above is the detailed content of Why Does Modifying a Python List During Iteration Lead to Unexpected Results?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


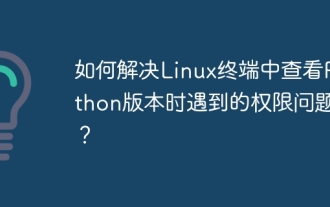
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
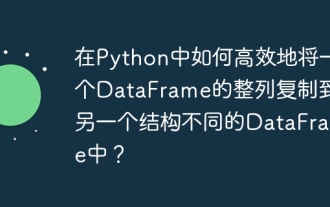
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
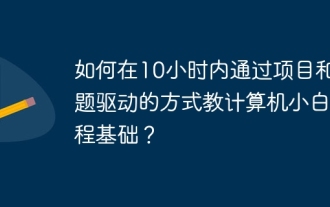
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
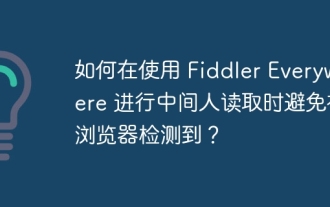
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
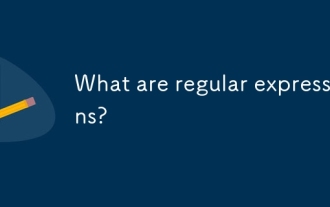
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
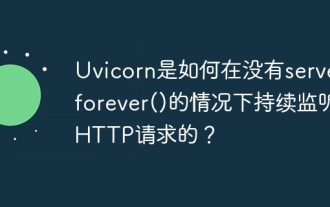
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
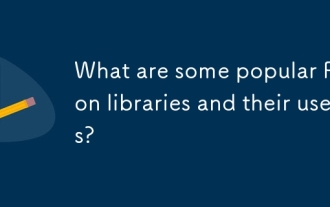
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
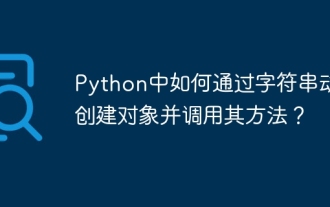
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
