How to Implement Scrolling in a Pygame Platformer?
In pygame, how can I add scrolling to a platformer?
In order to implement scrolling in a platformer game using pygame, you'll need to keep track of the player's position and the position of the camera.
-
Camera Class:
- Create a Camera class that will control the camera's movement.
- The Camera class should have a method to update the camera's position based on the player's position.
-
Player Position:
- Keep track of the player's position using the rect attribute of the player's sprite.
- Update the player's position based on user input (e.g., keyboard or joystick).
-
Camera Update:
- In the game loop, update the camera's position using the update() method of the Camera class.
- The update() method should take the player's position as an argument and adjust the camera's position accordingly.
-
Camera Offset:
- When drawing the game world, apply the camera's offset to the position of each object.
- This will make it appear as if the camera is moving through the world.
Here's an example of how this might look in your code:
import pygame class Camera: def __init__(self, width, height): self.width = width self.height = height self.x = 0 self.y = 0 def update(self, player): self.x = player.rect.x - self.width / 2 self.y = player.rect.y - self.height / 2 class Game: def __init__(self): self.screen = pygame.display.set_mode((800, 600)) self.clock = pygame.time.Clock() self.player = pygame.sprite.Sprite() self.player.rect = pygame.Rect(100, 100, 32, 32) self.camera = Camera(self.screen.get_width(), self.screen.get_height()) def run(self): while True: self.clock.tick(60) for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Update the player's position keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: self.player.rect.x -= 5 if keys[pygame.K_RIGHT]: self.player.rect.x += 5 if keys[pygame.K_UP]: self.player.rect.y -= 5 if keys[pygame.K_DOWN]: self.player.rect.y += 5 # Update the camera's position self.camera.update(self.player) # Draw the game world self.screen.fill((0, 0, 0)) self.screen.blit(self.player.image, self.player.rect - self.camera.pos) pygame.display.update() if __name__ == "__main__": game = Game() game.run()
The above is the detailed content of How to Implement Scrolling in a Pygame Platformer?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


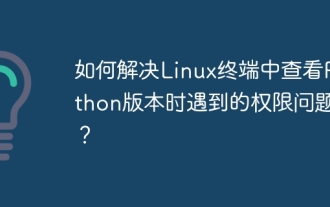
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
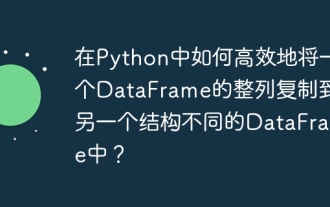
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
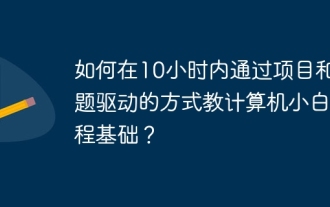
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
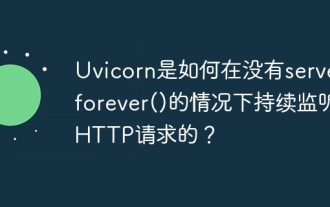
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
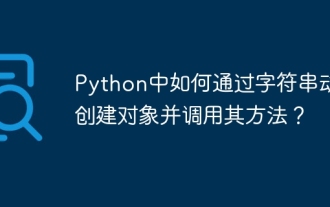
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
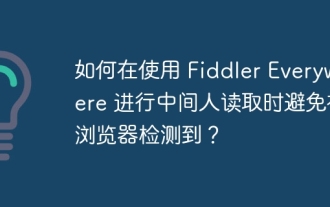
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
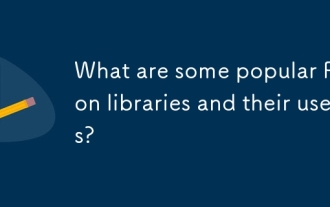
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
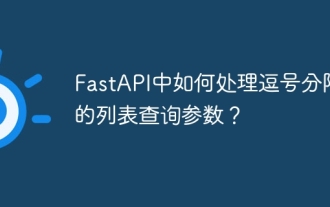
Fastapi ...
