How to Pass Multiple Return Values to a Variadic Function in Go?
Passing Multiple Return Values to a Variadic Function
Problem:
You have a Go function that returns two integers and want to print both values using string formatting within a fmt.Println() call. However, this approach is not supported by default in Go.
Solution:
While you can't directly pass multiple return values to fmt.Println(), you can use a trick to achieve the same result with fmt.Printf():
- Create a utility function, wrap(), that takes variable numbers of inputs and returns a slice of []interface{}.
- Call wrap() with the return values of your function, passing them as individual parameters.
- Pass the resulting slice to fmt.Printf() as the value for variadic parameter ....
Here's an example:
func wrap(vs ...interface{}) []interface{} { return vs } func twoInts() (int, int) { return 1, 2 } func main() { fmt.Printf("first= %d and second = %d", wrap(twoInts()...)...) }
This approach allows you to pass multiple return values to a variadic function, enabling you to print them using string formatting within fmt.Printf().
Note:
- fmt.Println() can still be used with functions that return at least one value, as it automatically assigns the excess return values to its variadic parameter.
- The wrap() utility function can be generalized to handle functions with any number of return values.
- For additional insights, refer to related questions on multiple values in single-value contexts and returning map-like 'ok' values in Go functions.
The above is the detailed content of How to Pass Multiple Return Values to a Variadic Function in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
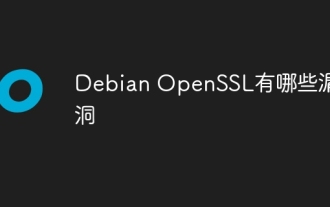
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
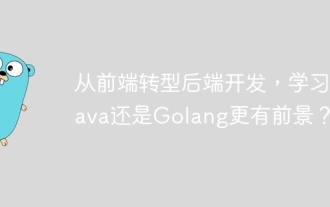
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
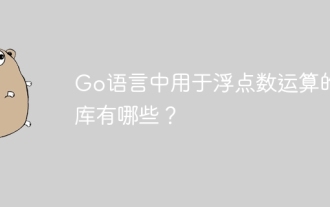
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
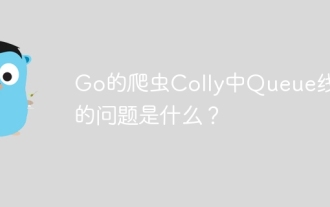
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
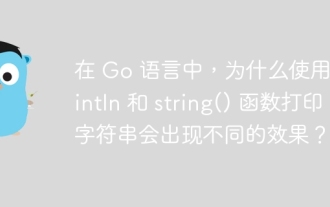
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
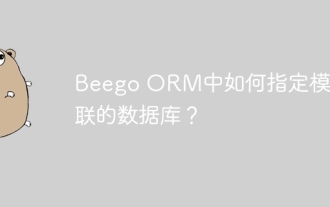
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
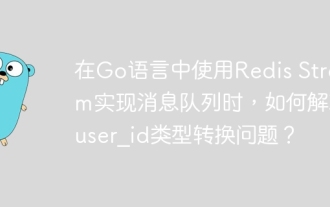
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
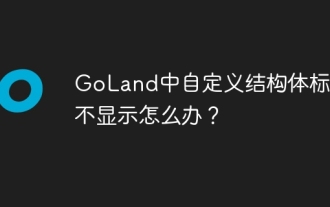
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
