How to Determine the Generic Type of a Java `List` Object?
How to Retrieve the Generic Type of a java.util.List
In Java, generics allow collections to store elements of a specific type, such as List
One approach to retrieving the generic type involves reflection, as demonstrated in the following code:
import java.lang.reflect.Field; import java.lang.reflect.ParameterizedType; import java.util.ArrayList; import java.util.List; public class Test { List<String> stringList = new ArrayList<>(); List<Integer> integerList = new ArrayList<>(); public static void main(String... args) throws Exception { Class<Test> testClass = Test.class; Field stringListField = testClass.getDeclaredField("stringList"); ParameterizedType stringListType = (ParameterizedType) stringListField.getGenericType(); Class<?> stringListClass = (Class<?>) stringListType.getActualTypeArguments()[0]; System.out.println(stringListClass); // class java.lang.String Field integerListField = testClass.getDeclaredField("integerList"); ParameterizedType integerListType = (ParameterizedType) integerListField.getGenericType(); Class<?> integerListClass = (Class<?>) integerListType.getActualTypeArguments()[0]; System.out.println(integerListClass); // class java.lang.Integer } }
This technique can be used to obtain the generic type of fields, parameter types, and return types of methods. However, it's important to note that if the generic type is within the same scope where it's declared, there is no inherent need to retrieve it since it's already known.
The above is the detailed content of How to Determine the Generic Type of a Java `List` Object?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




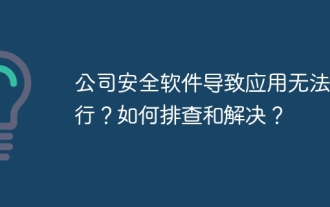
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
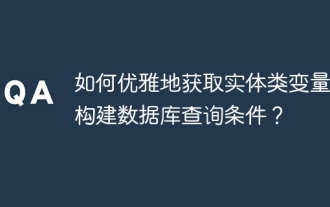
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
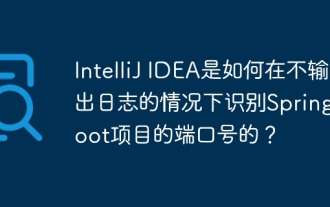
Start Spring using IntelliJIDEAUltimate version...
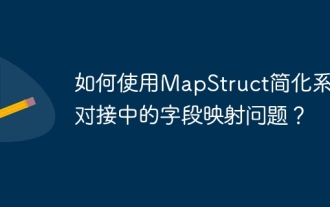
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
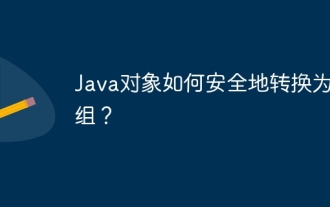
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
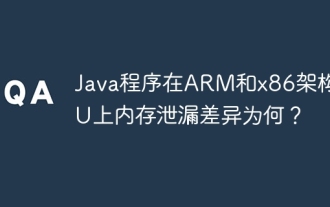
Analysis of memory leak phenomenon of Java programs on different architecture CPUs. This article will discuss a case where a Java program exhibits different memory behaviors on ARM and x86 architecture CPUs...
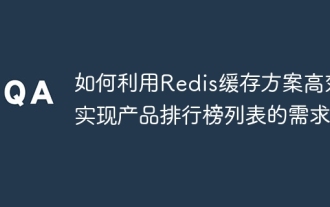
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
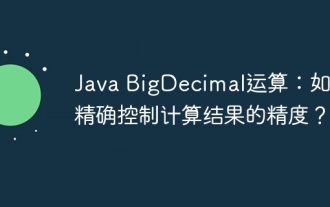
Java...
