How Can I Compare Function Pointers for Equality in Go?
Function Pointer Equality in Go
In Go, comparing two non-nil function pointers for equality using the standard pointer equality operator == has become invalid in recent versions. This is a departure from the pre-Go1 behavior where function pointers could be compared for identity.
Rationale for Not Allowing Pointer Equality of Functions
The prohibition on comparing function pointers for equality was motivated by the following reasons:
- Performance: Functions may be implemented as closures, which do not capture variables from their environment. Allowing identity comparisons would require the runtime to create a new closure for each function, resulting in performance overhead.
- Clarity: To avoid mixing equality and identity, the Go1 operators == and != only compare values for equivalence, not identity. This ensures a consistent approach throughout the language.
Achieving Pointer Equality of Functions
While direct pointer comparison of functions is no longer allowed, there are alternative approaches to achieve the desired behavior:
- Assign Unique Variables: Declare separate variables for each function and compare their addresses:
package main import "fmt" func F1() {} func F2() {} var F1_ID = F1 // Create a *unique* variable for F1 var F2_ID = F2 // Create a *unique* variable for F2 func main() { f1 := &F1_ID // Take the address of F1_ID f2 := &F2_ID // Take the address of F2_ID fmt.Println(f1 == f1) // Prints true fmt.Println(f1 == f2) // Prints false }
- Use Reflection: The reflect package provides low-level access to object information, including function pointers. You can use it to compare function identities:
package main import "fmt" import "reflect" func SomeFun() {} func AnotherFun() {} func main() { sf1 := reflect.ValueOf(SomeFun) sf2 := reflect.ValueOf(SomeFun) fmt.Println(sf1.Pointer() == sf2.Pointer()) // Prints true af1 := reflect.ValueOf(AnotherFun) fmt.Println(sf1.Pointer() == af1.Pointer()) // Prints false }
Note: Using reflect relies on undefined behavior. It does not guarantee consistency across platforms or Go versions.
The above is the detailed content of How Can I Compare Function Pointers for Equality in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
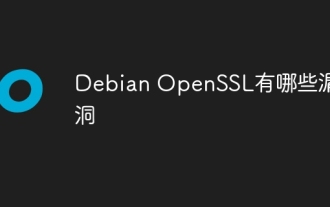
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
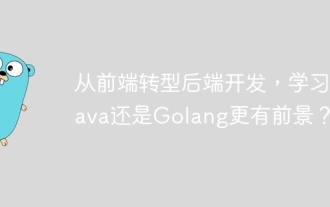
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
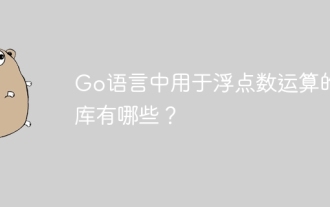
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
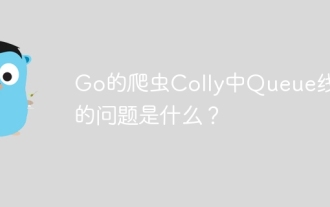
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
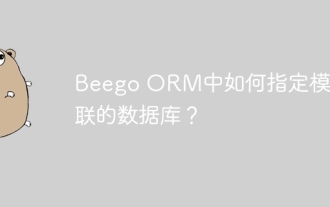
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
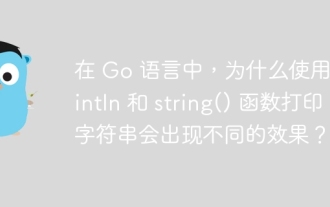
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
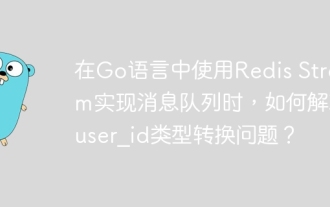
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
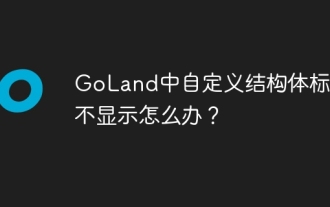
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
