


Functional Programming in Go with IBM fp-go: Error Handling Made Explicit
Functional programming (FP) principles are gaining popularity in modern software development due to their emphasis on immutability, composability, and explicitness. While Go is traditionally an imperative language, the fp-go library, developed by IBM, introduces FP abstractions such as Option, Either, Fold, and utilities for functional composition. In this article, we will explore how to use fp-go to handle errors explicitly, define function signatures with multiple error types, and build a real-world CRUD API example demonstrating these concepts.
Why Functional Error Handling?
Error handling is crucial for building reliable software. Traditional Go error handling relies on returning error values, which can be unintentionally ignored or mishandled. Functional error handling introduces abstractions like:
- Option: Represents optional values, akin to Some and None in other FP languages.
- Either: Encapsulates a value that can either be a Right (success) or Left (failure), making error propagation explicit.
- Tagged Unions: Allow function signatures to clearly define possible error types.
- Composition: Enables chaining operations while handling errors naturally.
Let’s dive into these concepts and see how fp-go facilitates them in Go.
Getting Started with fp-go
First, add fp-go to your Go project:
go get github.com/IBM/fp-go
Import the necessary modules:
import ( either "github.com/IBM/fp-go/either" option "github.com/IBM/fp-go/option" )
Option: Handling Optional Values
Option represents a value that may or may not exist. It is either Some(value) or None.
Example: Parsing an Integer
func parseInt(input string) option.Option[int] { value, err := strconv.Atoi(input) if err != nil { return option.None[int]() } return option.Some(value) } func main() { opt := parseInt("42") option.Fold( func() { fmt.Println("No value") }, func(value int) { fmt.Printf("Parsed value: %d\n", value) }, )(opt) }
Key Takeaways:
- Option eliminates nil values.
- Fold is used to handle both cases (Some or None).
Either: Handling Errors Explicitly
Either represents a computation that can result in two possibilities:
- Left: Represents an error.
- Right: Represents a successful result.
Example: Safe Division
type MathError struct { Code string Message string } func safeDivide(a, b int) either.Either[MathError, int] { if b == 0 { return either.Left(MathError{Code: "DIV_BY_ZERO", Message: "Cannot divide by zero"}) } return either.Right(a / b) } func main() { result := safeDivide(10, 0) either.Fold( func(err MathError) { fmt.Printf("Error [%s]: %s\n", err.Code, err.Message) }, func(value int) { fmt.Printf("Result: %d\n", value) }, )(result) }
Key Takeaways:
- Either separates success and failure paths.
- Fold simplifies handling both cases in one place.
Function Signatures with Multiple Error Types
Real-world applications often need to handle multiple types of errors. By using tagged unions, we can define explicit error types.
Example: Tagged Union for Errors
go get github.com/IBM/fp-go
Benefits:
- Tagged unions make errors self-documenting.
- Explicit types reduce ambiguity in error handling.
Real-World Example: CRUD API
Let’s implement a simple CRUD API with explicit error handling using Either.
Model and Error Definitions
import ( either "github.com/IBM/fp-go/either" option "github.com/IBM/fp-go/option" )
Repository Layer
func parseInt(input string) option.Option[int] { value, err := strconv.Atoi(input) if err != nil { return option.None[int]() } return option.Some(value) } func main() { opt := parseInt("42") option.Fold( func() { fmt.Println("No value") }, func(value int) { fmt.Printf("Parsed value: %d\n", value) }, )(opt) }
Service Layer
type MathError struct { Code string Message string } func safeDivide(a, b int) either.Either[MathError, int] { if b == 0 { return either.Left(MathError{Code: "DIV_BY_ZERO", Message: "Cannot divide by zero"}) } return either.Right(a / b) } func main() { result := safeDivide(10, 0) either.Fold( func(err MathError) { fmt.Printf("Error [%s]: %s\n", err.Code, err.Message) }, func(value int) { fmt.Printf("Result: %d\n", value) }, )(result) }
Controller
type AppError struct { Tag string Message string } const ( MathErrorTag = "MathError" DatabaseErrorTag = "DatabaseError" ) func NewMathError(msg string) AppError { return AppError{Tag: MathErrorTag, Message: msg} } func NewDatabaseError(msg string) AppError { return AppError{Tag: DatabaseErrorTag, Message: msg} } func process(a, b int) either.Either[AppError, int] { if b == 0 { return either.Left(NewMathError("Division by zero")) } return either.Right(a / b) } func main() { result := process(10, 0) either.Fold( func(err AppError) { fmt.Printf("Error [%s]: %s\n", err.Tag, err.Message) }, func(value int) { fmt.Printf("Processed result: %d\n", value) }, )(result) }
Conclusion
Using fp-go in Go, we can:
- Model errors explicitly using Either.
- Represent optional values with Option.
- Handle multiple error types via tagged unions.
- Build maintainable and composable APIs.
These patterns make your Go code more robust, readable, and functional. Whether you’re building a CRUD API or complex business logic, fp-go empowers you to handle errors cleanly and consistently.
The above is the detailed content of Functional Programming in Go with IBM fp-go: Error Handling Made Explicit. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










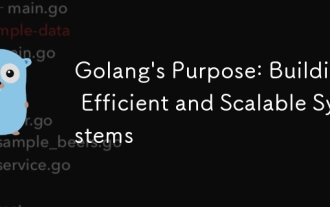
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
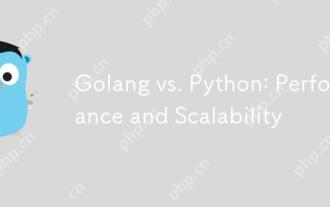
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
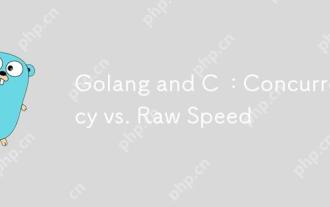
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
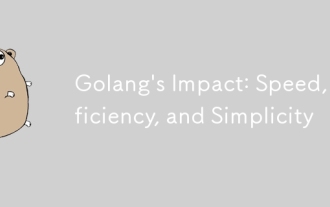
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
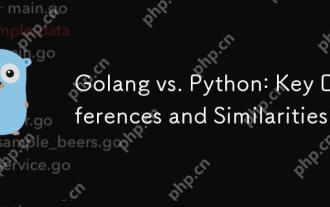
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
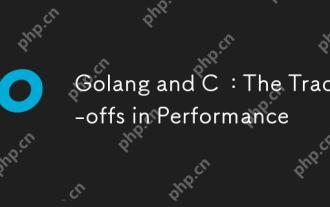
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
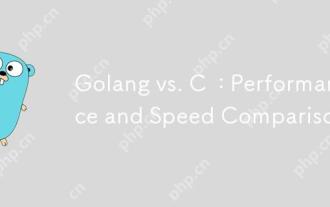
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
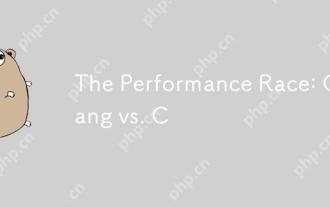
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
