


How Can I Work with Go Maps Having the Same Key Type but Different Value Types?
Extending Map Usages with Heterogeneous Value Types
Maps in Go provide a powerful data structure for managing key-value pairs. However, when working with collections of maps possessing the same key type but varying value types, the lack of built-in support can become a limitation.
Consider the following code snippet:
func useKeys(m map[string]interface{}) { //something with keys here }
The intention is to write a generic function that operates on the keys of any map with a string key type, regardless of the value type. However, attempts like the one above fail due to the type mismatch.
The fundamental challenge lies in the absence of covariance in Go's map and slice types. Unlike generic languages, Go does not support covariantly subtyping, meaning that a map with a derived key or value type is not compatible with a map with a base key or value type.
Practical Approaches
While there is no elegant solution to this problem, there are practical workarounds:
Explicit Type Handling:
One approach is to create separate functions for each specific map type. For example:
func useKeysInts(m map[string]int) { //operations on string keys and int values } func useKeysDoubles(m map[string]double) { //operations on string keys and double values }
Reflection-Based Approach:
Reflection provides a more dynamic solution, allowing you to examine and manipulate the map's structure and contents. The following function uses reflection to extract the keys from any map:
func useKeysReflect(m interface{}) { v := reflect.ValueOf(m) if v.Kind() != reflect.Map { fmt.Println("not a map!") return } keys := v.MapKeys() fmt.Println(keys) }
This approach is useful when you need to perform operations on the keys of a map of unknown or dynamically generated types.
It's worth noting that the reflection-based approach may incur some runtime overhead due to the additional processing required.
The above is the detailed content of How Can I Work with Go Maps Having the Same Key Type but Different Value Types?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


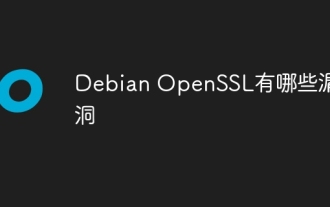
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
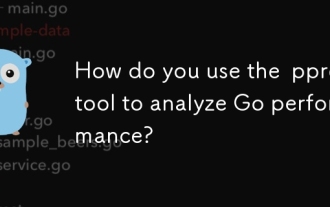
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
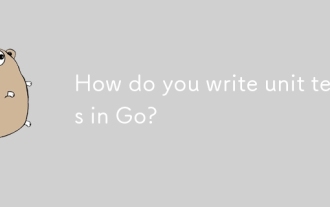
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
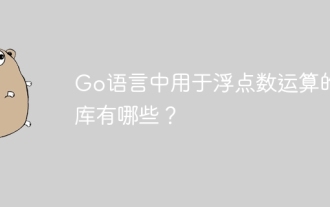
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
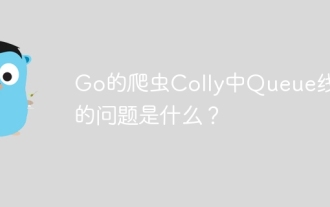
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
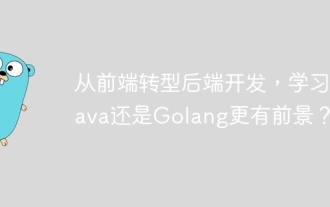
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
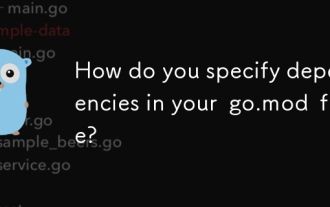
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
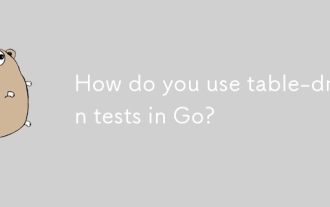
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
