


How Can I Efficiently Load and Display Large Text Files in Swing Applications?
Loading and Displaying Large Text Files
In Swing applications, displaying large text files can be challenging due to performance issues. For small amounts of data, a Document and JTextComponent may suffice. However, for larger files in the 10-100 megabyte range, a more practical alternative is necessary.
Solution: JTable and SwingWorker
To handle large text files effectively, consider the following approach:
- Load the file in the background using SwingWorker to avoid blocking the event dispatch thread.
- Instead of using a Document, update a TableModel and display the lines of text in the rows of a JTable.
This approach offers several advantages:
- Immediate display: Results appear immediately, reducing perceived latency.
- Scalability: JTable uses the flyweight pattern, which scales well for large amounts of data.
- Flexibility: You can parse the input on the fly and create an arbitrary column structure.
- Functionality: JTable provides sorting, filtering, and TablePopupEditor capabilities.
Example: Using JTable and SwingWorker
The following code snippet demonstrates how to use JTable and SwingWorker to load and display a large text file:
// SwingWorker to load the file in the background private LogWorker lw = new LogWorker(new File(NAME), model); // PropertyChangeListener to update the progress bar lw.addPropertyChangeListener((e) -> { SwingWorker.StateValue s = (SwingWorker.StateValue) e.getNewValue(); jpb.setIndeterminate(s.equals(SwingWorker.StateValue.STARTED)); }); lw.execute();
Additional Considerations
- For optimal performance, extend AbstractTableModel and manage a List
or List . - To display intermediate progress, modify the code as shown in the link provided in the answer.
The above is the detailed content of How Can I Efficiently Load and Display Large Text Files in Swing Applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










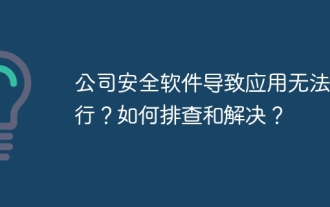
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
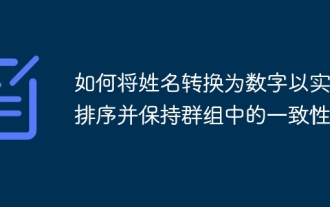
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
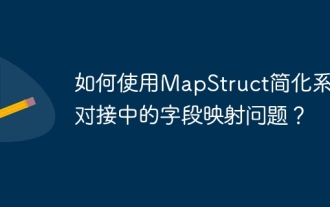
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
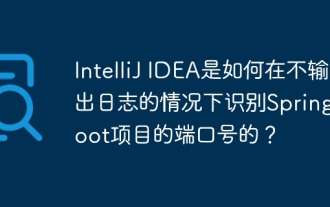
Start Spring using IntelliJIDEAUltimate version...
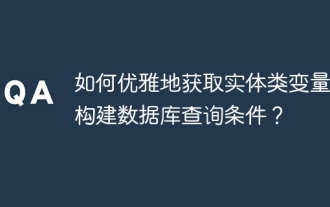
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
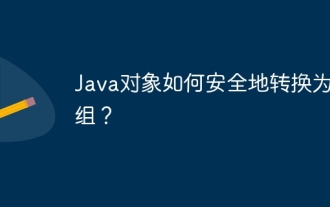
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
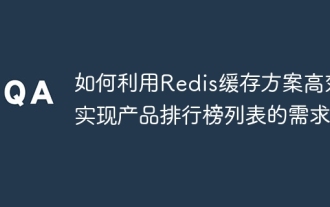
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
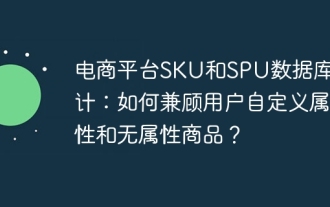
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
