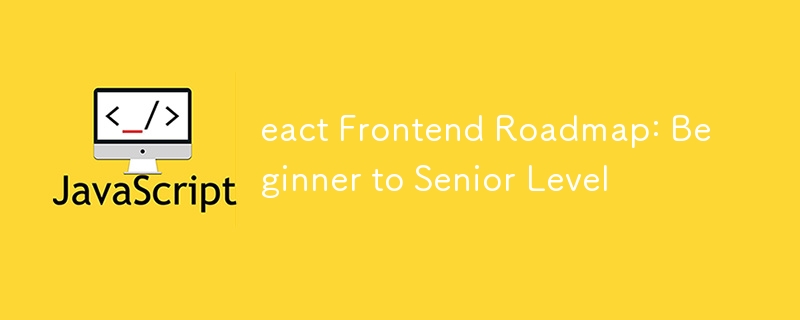
Here’s a React 19 and Next.js 15 roadmap to guide a developer’s journey from a fresher to a senior developer. The roadmap is divided into stages, focusing on skills, tools, and concepts relevant to each level of expertise.
1. Fresher (Entry-Level)
Goals: Master the basics of React and Next.js. Build small projects.
Key Learning Areas:
-
React Basics:
- JSX, Components, Props, State, and Events.
- Functional Components and Hooks (useState, useEffect).
- Conditional Rendering and Lists.
-
Next.js Basics:
- Pages and Routing (pages/ directory, dynamic routes).
- Static Site Generation (SSG) and Server-Side Rendering (SSR).
- API Routes (/api).
-
Styling:
- CSS Modules, Tailwind CSS, or Styled Components.
-
Tooling:
- Basic usage of npm/yarn and version control with Git.
-
Practice:
- Build a personal portfolio site or a blog with SSG.
Suggested Projects:
- Todo App.
- Weather App (using API routes).
- Blog with Markdown (SSG and dynamic routes).
Here all Public API free for practising: https://github.com/public-apis/public-apis
---
2. Junior Developer
Goals: Work on real-world projects and understand advanced React and Next.js concepts.
Key Learning Areas:
-
React Advanced Concepts:
- Context API for state management.
- Optimizations (React.memo, lazy loading, suspense).
-
Next.js Features:
- Middleware for route protection and customization.
- Image Optimization.
- Incremental Static Regeneration (ISR).
- Handling Data Fetching (getStaticProps, getServerSideProps, getInitialProps).
-
State Management:
- Redux Toolkit, Zustand, or Jotai.
-
Forms and Validation:
- Formik or React Hook Form.
- Yup for validation.
-
Authentication:
- NextAuth or Auth0 integration.
-
Tooling:
- Linters (ESLint), Formatters (Prettier).
- Unit Testing with Jest and React Testing Library.
-
Best Practices:
- Folder structure and clean coding standards.
-
Practice:
- Collaborate on open-source or team projects.
Suggested Projects:
- E-commerce Website with authentication and dynamic product pages.
- Admin Dashboard with client/server data fetching.
- Blog with comments and user authentication.
3. Mid-Level Developer
Goals: Lead feature development, optimize performance, and start mentoring juniors.
Key Learning Areas:
-
React Advanced Patterns:
- Higher Order Components (HOCs) and Render Props.
- Compound Components and Controlled vs Uncontrolled Components.
-
Next.js Optimization:
- Improve page performance using middleware and caching.
- Optimize bundle size and reduce server response time.
-
Advanced State Management:
- React Query or SWR for data fetching and caching.
-
Fullstack Development:
- Work with backends like NestJS, Node.js, or serverless functions.
-
Testing:
- Integration testing with Cypress.
- Write end-to-end tests.
-
Deployment and Monitoring:
- Vercel for deployments.
- Monitoring tools like Sentry or LogRocket.
-
Mentorship:
- Code reviews and pair programming with juniors.
Suggested Projects:
- Multi-role SaaS platform.
- CMS-like application with dynamic data and admin features.
- Real-time chat app (using WebSocket or Firebase).
4. Senior Developer
Goals: Architect applications, lead teams, and focus on scalability and maintainability.
Key Learning Areas:
-
System Design:
- Architecting scalable React and Next.js applications.
- Optimize API calls and caching.
- Use microservices or serverless architecture.
-
Advanced Next.js Features:
- Internationalization (i18n).
- Custom server handling with Express or Fastify.
- Custom Webpack configurations for advanced use cases.
-
Performance Tuning:
- Analyze and fix bottlenecks using Lighthouse or WebPageTest.
- Improve user experience with progressive web apps (PWAs).
-
Collaboration and Leadership:
- Guide teams in design patterns and best practices.
- Lead technical discussions and decisions.
-
DevOps and CI/CD:
- Automate testing, builds, and deployments with GitHub Actions or Jenkins.
-
Open Source Contributions:
- Contribute to Next.js or React projects.
- Create reusable libraries and publish them (e.g., on npm).
Suggested Projects:
- High-performance Progressive Web Application (PWA).
- Enterprise-grade dashboard with real-time analytics.
- Complex multilingual e-commerce application.
Learning Resources:
-
React:
- React Official Docs
- Egghead.io Courses on React.
-
Next.js:
- Next.js Official Docs
- Learn with Vercel Tutorials.
-
State Management:
- Redux Toolkit, Zustand, or React Query docs.
-
Books and Videos:
- "React Design Patterns and Best Practices."
- Frontend Masters courses.
This roadmap equips developers at every stage to progressively enhance their skills, making them capable of handling increasingly complex challenges.
The above is the detailed content of eact Frontend Roadmap: Beginner to Senior Level. For more information, please follow other related articles on the PHP Chinese website!