From zero to Software Engineer
? From zero to Software Engineer in 11 steps ??
If you are starting your path towards software engineering, here is a detailed guide to master the basics, grow in your career and become a professional with solid knowledge, using Python as a base language.
It is impossible to cover the entire roadmap to becoming a software engineer in detail in a single article. Therefore, at each stage links to specialized resources that address specific topics are included.
1. ? Set up your development environment
Before writing your first line of code, it is crucial to have the environment ready.
- Code Editor:
Download and configure Visual Studio Code (lightweight and flexible) or PyCharm (powerful for Python).
- Python Installation:
Visit python.org to install the latest version.
If you are using Windows, Set the PATH on your system to run Python from the terminal.
- Installation of additional tools:
Install pip (Python package manager) to easily install libraries.
Set up a virtual environment (venv) to isolate projects.
- ⏩Extra:
Learn how to use Jupyter Notebook to explore interactive code.
2. ? Master the fundamentals of programming with Python
Learning the fundamentals will give you the tools to solve any problem. Focus on:
- Basic syntax: variables, input/output (print, input).
- Control structures: if-else, for and while loops.
- Functions: definition, arguments, return.
- Error Handling: Use try-except blocks to prevent your program from breaking or generating unhandled errors.
- Collections: lists, dictionaries, tuples and sets.
- ? Exercise: Create a basic calculator and a program that sorts a list.
? Resource: Python Basics - FreeCodeCamp.
3. ? Learn Object Oriented Programming (OOP)
OOP is key in software engineering, as it allows you to organize and reuse your code. Learn:
Classes and Objects:
python
Copy code
class Person:
def init(self, name):
self.name = name
p = Person("Ana")
print(p.name)
Encapsulation: Protect attributes with _private or __very_private.
Inheritance: Extend existing classes without rewriting everything.
Polymorphism: Methods with different behaviors.
? Practical exercise: Create a Vehicle class with attributes and subclasses such as Car or Motorcycle.
4. ?️ Learn to use Git and GitHub
Every software engineer must master version control.
Install Git:
Configure your name and email with git config.
Learn the basic Commands:
git init: Initializes a repository.
git add .: Add changes.
git commit -m "Message": Save the change.
git push: Upload your code.
? Practical project: Upload a Python script to your first repository. Make changes and view history with git log.
5. ? Develop web applications with Python
Python is excellent for backend development. Starts with:
- Flask (light and fast): Learn to create routes (@app.route) and responses.
- Django (complete and robust): Generate a project structure with django-admin startproject. Learn about views, models and templates.
- ? Practical exercise: Create a basic server that displays “Hello World” and deploy your app to Heroku or Render.
6. ? Master databases
Data management is essential in software. Learn:
- SQL: Use SELECT, INSERT, UPDATE and DELETE commands.
- SQLite/PostgreSQL: Configure your local database.
- ORM with Django/SQLAlchemy: Manage databases using Python code.
- ? Practical project: Create a database that stores pending tasks and access it from your Flask app.
7. ? Improve your logic with algorithms and data structures
Problem solving improves your critical thinking. Master:
Search and sorting algorithms: binary search, merge sort.
Data structures: lists, queues, stacks, trees and graphs.
? Daily Practice:
Solve problems in LeetCode and HackerRank.
Break down big problems into smaller solutions.
8. ? Learn about Testing and code quality
Write robust code using automated tests:
Pytest: Simple and powerful framework for testing.
Doctest: Add tests in your code documentation.
Basic test example with pytest:
def sum(a, b):
return a b
def test_sum():
assert sum(2, 3) == 5
9. ? Learn about APIs and microservices
The creation of APIs allows communication between applications.
- RESTful APIs: Use Flask or FastAPI to create routes and return data in JSON.
- API consumption: Use libraries as requests.
- ? Practical exercise: Create an API that sends weather data using external data such as OpenWeatherMap.
10. ? Build real projects and create a portfolio
Apply everything learned in practical projects:
- CRUD Application: Management of tasks, users or notes.
- Automated script: For example, a bot that checks your email.
- REST API: Publish useful data and documentation (with Swagger).
- Web Portfolio: Create a portfolio using Flask or Django.
- ? Resource: Use GitHub Pages to deploy projects and show your code.
11. ? Apply and get a job
Prepare your CV and start applying for vacancies. Practice makes perfect; little by little you will improve in the interviews.
To look for a job in the IT sector, you can use a specialized portal for IT profiles such as:
- We Work Remotely
- Getonbrd
- Hireline
- Findjobit
- Wellfound
? What's next?
Once you execute these 11 steps, consider exploring:
- Cloud development (AWS, Azure).
- DevOps and automation with Docker and CI/CD.
- Distributed systems.
? Start now and advance step by step! Each line of code brings you closer to your goal: becoming a software engineer. ?
The above is the detailed content of From zero to Software Engineer. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




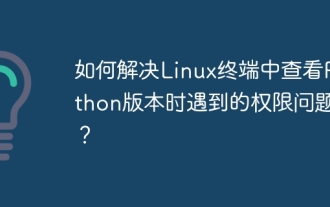
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
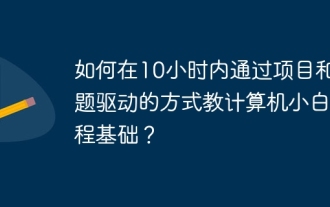
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
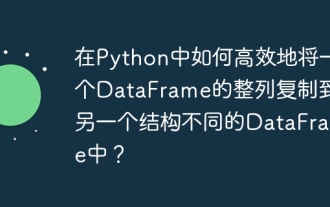
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
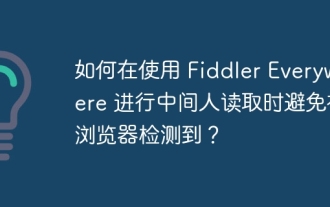
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
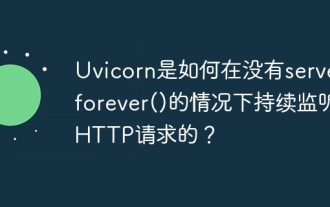
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
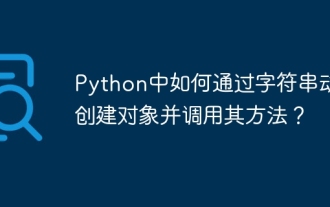
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
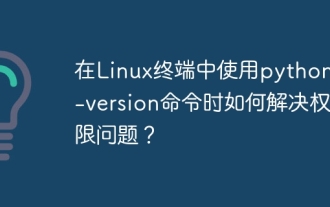
Using python in Linux terminal...
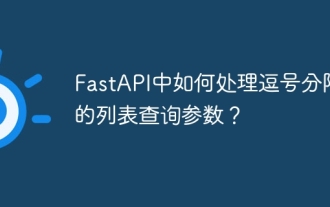
Fastapi ...
