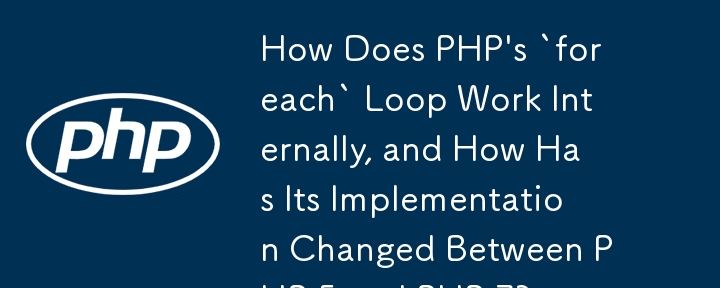
How does PHP 'foreach' actually work?
PHP's foreach iterates over arrays, objects, and Traversable objects.
Arrays:
- Foreach iterates over an array's keys and values.
- Modifications during iteration can lead to unexpected behavior.
- In PHP 5, foreach uses an internal array pointer (IAP) that can be shared between multiple loops, leading to complex behavior.
- In PHP 7, foreach uses external, safe hashtable iterators, eliminating the need for the IAP.
Objects:
- Foreach iterates over an object's properties and values.
- Modifications during iteration are reflected in the loop.
- This is due to PHP's by-handle passing semantics, which make objects behave like references.
Traversable Objects:
- Foreach essentially provides a syntactic shortcut for traversing Traversable objects.
Examples:
- Duplication: PHP 5 duplicates arrays before iterating if the array is not a reference and has a reference count greater than 1. PHP 7 avoids this duplication whenever possible.
- Position Advancement Order: Foreach advances the array pointer before the loop body runs, so it works on element i 1 while the loop body is still on element i.
- Modification During Iteration: Foreach skips removed elements in PHP 5 due to its reliance on the IAP. In PHP 7, foreach continues iterating even after element removal.
- Reset() and Each(): In PHP 5, HashPointer backup and restore mechanisms prevent reset() from affecting foreach. In PHP 7, reset() works independently from foreach.
- Substituted Entities: PHP allows substitution of the iterated entity during the loop (e.g., starting with an array and switching to an object).
In Summary:
PHP's foreach iterates over arrays and objects efficiently, providing convenient access to their elements. Understanding its implementation details (especially the differences between PHP 5 and PHP 7) can help you avoid unexpected behavior when modifying arrays during iteration.
The above is the detailed content of How Does PHP's `foreach` Loop Work Internally, and How Has Its Implementation Changed Between PHP 5 and PHP 7?. For more information, please follow other related articles on the PHP Chinese website!