


How can I efficiently merge multiple DataTables with varying schemas into a single DataTable in C#?
Combining Multiple DataTables with Variable Schemas into a Single Table
To address the issue of combining multiple DataTables into a single table, we recommend an efficient method that effectively handles the varying column structures and row alignment.
Quick Solution
The following C# code snippet provides a straightforward approach:
public static DataTable MergeTables(IList<DataTable> tables, string primaryKeyColumn) { if (!tables.Any()) throw new ArgumentException("Tables must not be empty", "tables"); if (primaryKeyColumn != null) foreach (DataTable t in tables) if (!t.Columns.Contains(primaryKeyColumn)) throw new ArgumentException("All tables must have the specified primarykey column " + primaryKeyColumn, "primaryKeyColumn"); if (tables.Count == 1) return tables[0]; DataTable table = new DataTable("TblUnion"); table.BeginLoadData(); // Turns off notifications, index maintenance, and constraints while loading data foreach (DataTable t in tables) { table.Merge(t); // same as table.Merge(t, false, MissingSchemaAction.Add); } table.EndLoadData(); if (primaryKeyColumn != null) { // join rows with repeating primary key columns var pkGroups = table.AsEnumerable() .GroupBy(r => r[primaryKeyColumn]); var dupGroups = pkGroups.Where(g => g.Count() > 1); foreach (var grpDup in dupGroups) { // use first row and modify it DataRow firstRow = grpDup.First(); foreach (DataColumn c in table.Columns) { if (firstRow.IsNull(c)) { DataRow firstNotNullRow = grpDup.Skip(1).FirstOrDefault(r => !r.IsNull(c)); if (firstNotNullRow != null) firstRow[c] = firstNotNullRow[c]; } } // remove all but first row var rowsToRemove = grpDup.Skip(1); foreach(DataRow rowToRemove in rowsToRemove) table.Rows.Remove(rowToRemove); } } return table; }
Usage
To utilize the MergeTables method, follow these steps:
- Define a list of input DataTables.
- Optionally, specify the name of the primary key column if the tables share a common primary key.
- Call the MergeTables method with the list of tables and the primary key column, if applicable.
- The method returns a single DataTable with the merged data, handling any discrepancies in column structures and row alignment.
Example
Consider the following sample DataTables:
DataTable1 (Columns: c1, c2, c3, c4) | c1 | c2 | c3 | c4 | |---|---|---|---| | 1 | 8500 | abc | A | | 2 | 950 | cde | B | | 3 | 150 | efg | C | | 4 | 850 | ghi | D | | 5 | 50 | ijk | E | DataTable2 (Columns: c1, c5, c6, c7) | c1 | c5 | c6 | c7 | |---|---|---|---| | 1 | 7500 | klm | F | | 2 | 900 | mno | G | | 3 | 150 | opq | H | | 4 | 850 | qrs | I | | 5 | 50 | stu | J | DataTable3 (Columns: c1, c8, c9, c10) | c1 | c8 | c9 | c10 | |---|---|---|---| | 1 | 7500 | uvw | K | | 2 | 900 | wxy | L | | 3 | 150 | yza | M | | 4 | 850 | ABC | N | | 5 | 50 | CDE | O |
To merge these three tables, we can call the MergeTables method as follows:
var tables = new[] { DataTable1, DataTable2, DataTable3 }; DataTable mergedTable = MergeTables(tables, "c1");
The mergedTable will contain all the rows from the three input tables, with the columns aligned by the common primary key column "c1". Any missing values in a row will be populated from the first non-null row with the same primary key value.
The above is the detailed content of How can I efficiently merge multiple DataTables with varying schemas into a single DataTable in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










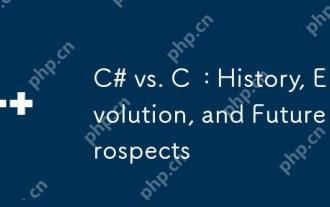
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
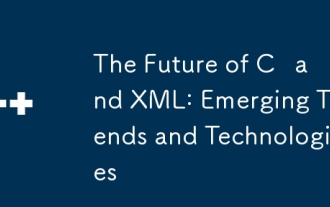
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
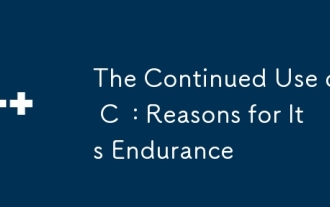
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
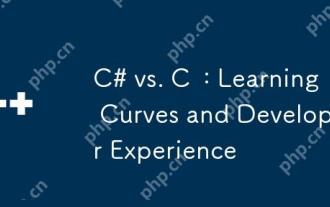
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
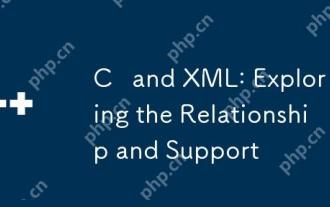
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
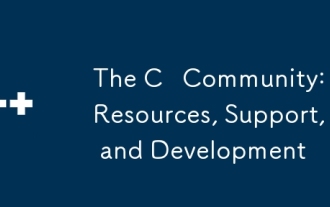
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
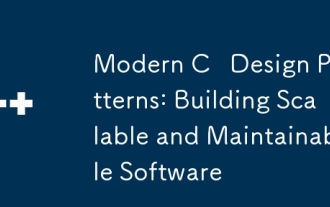
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
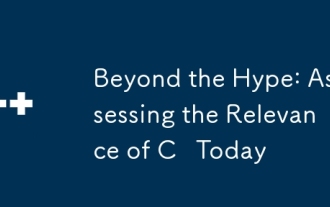
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
