How Can I Efficiently Convert Maps to Structs in Go?
Converting Maps to Structs in Go: Efficient Approaches
Filling structs with data from maps in Go can be a common task, and using an intermediary JSON conversion can feel inefficient. Fortunately, there are more efficient ways to accomplish this transformation.
One highly recommended approach is to leverage the versatile "mapstructure" package from Mitchell Hashimoto. With this package, you can simply invoke:
import "github.com/mitchellh/mapstructure" mapstructure.Decode(myData, &result)
This elegant syntax will decode the map myData into the struct result with minimal fuss.
If you prefer a more DIY approach, you can follow the comprehensive solution outlined in the code snippet below:
func SetField(obj interface{}, name string, value interface{}) error { // Get a handle on the struct value and field structValue := reflect.ValueOf(obj).Elem() structFieldValue := structValue.FieldByName(name) // Check validity and permissions for the operation if !structFieldValue.IsValid() { return fmt.Errorf("No such field: %s in obj", name) } if !structFieldValue.CanSet() { return fmt.Errorf("Cannot set %s field value", name) } // Match field types for assignment structFieldType := structFieldValue.Type() val := reflect.ValueOf(value) if structFieldType != val.Type() { return errors.New("Provided value type didn't match obj field type") } // Update the field value within the struct instance structFieldValue.Set(val) return nil } type MyStruct struct { Name string Age int64 } func (s *MyStruct) FillStruct(m map[string]interface{}) error { // Iterate over map keys and values, setting corresponding struct fields for k, v := range m { err := SetField(s, k, v) if err != nil { return err } } return nil } func main() { myData := make(map[string]interface{}) myData["Name"] = "Tony" myData["Age"] = int64(23) result := &MyStruct{} err := result.FillStruct(myData) if err != nil { fmt.Println(err) } fmt.Println(result) }
This code will meticulously handle field lookups, type checks, and value assignments to achieve the conversion from map to struct.
The above is the detailed content of How Can I Efficiently Convert Maps to Structs in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


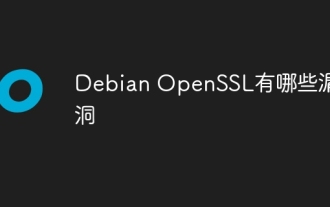
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
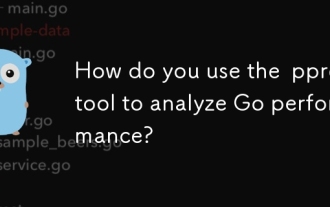
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
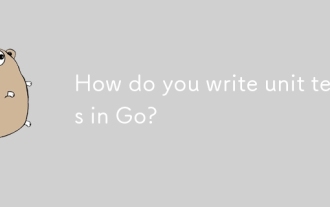
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
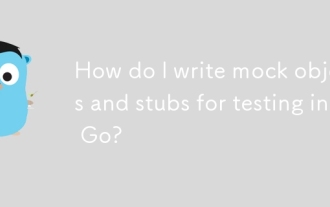
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
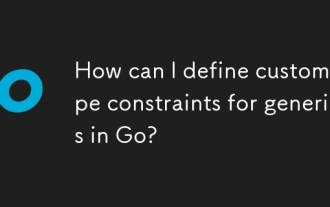
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
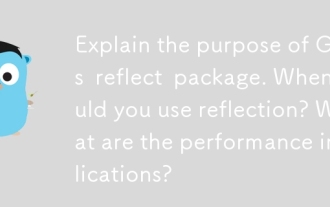
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
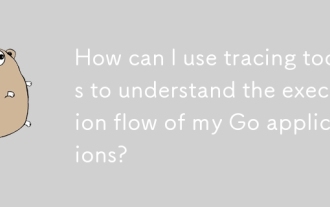
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
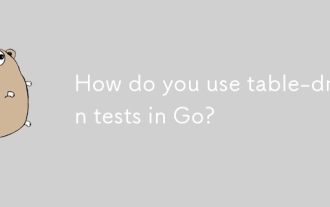
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
