


How to Efficiently Fill a DataSet with Multiple Tables Using a DataReader?
Filling a DataSet with Multiple Tables Using a DataReader
When working with a DataSet that contains multiple tables with one-to-many relationships, it's possible to fill it using a DataReader. However, the default approach of using a single DataReader may not capture data from all tables.
To overcome this limitation, you can use the following approach:
using System.Data; using System.Data.SqlClient; using System.IO; namespace SampleApp { public class DataSetWithTables { private SqlConnection connection; public DataSet SelectOne(int id) { DataSet result = new DataSet(); string query = @"select * from table1; select * from table2 where table1_id = @ID;"; using (connection = new SqlConnection("ConnectionString")) { connection.Open(); using (SqlCommand command = new SqlCommand(query, connection)) { command.Parameters.AddWithValue("ID", id); using (SqlDataReader reader = command.ExecuteReader()) { DataTable table1 = new DataTable("Table1"); DataTable table2 = new DataTable("Table2"); table1.Load(reader); if (reader.NextResult()) { table2.Load(reader); } result.Tables.Add(table1); result.Tables.Add(table2); } } connection.Close(); } return result; } } }
In this approach:
- We define a query that includes two SELECT statements, one for each table.
- We use a SqlCommand with parameters to execute the query.
- We execute the ExecuteReader command and use reader.NextResult() to load data into the second table.
- We create two DataTable objects to represent our tables and load the data into them.
- We add the DataTable objects to the DataSet and close the connection.
The above is the detailed content of How to Efficiently Fill a DataSet with Multiple Tables Using a DataReader?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


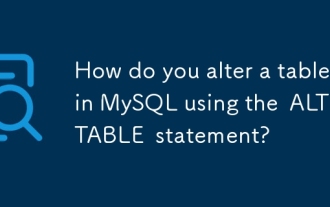
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
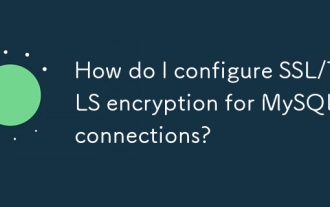
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
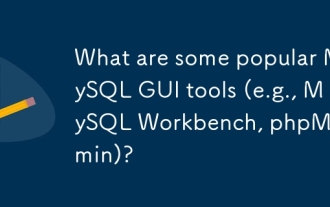
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
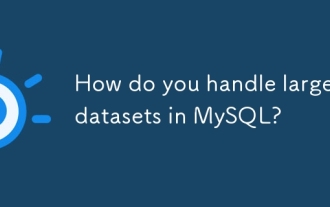
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
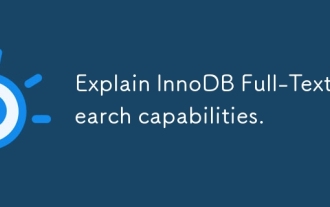
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
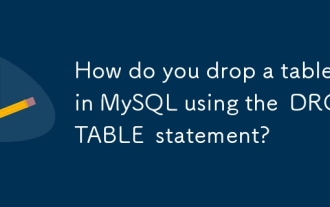
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
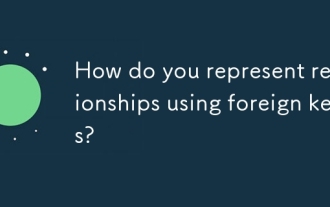
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
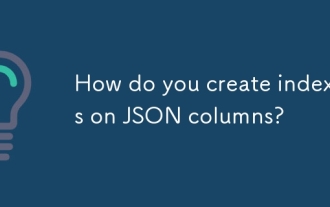
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
