


How to Find Rows with the Maximum Count in Pandas GroupBy Operations?
Get Rows with Maximum Count in Pandas Groups
Problem:
How to identify rows with the highest value for the 'count' column in a pandas DataFrame when grouping by multiple columns?
Solution:
Step 1: Find Maximum Count for Each Group
To determine the maximum count for each group, use the groupby() and max() functions:
max_counts = df.groupby(['Sp', 'Mt'])['count'].max()
This will create a Series containing the maximum count for each group.
Step 2: Identify Rows with Maximum Count
To get the indices of the rows with maximum count in the original DataFrame, use the transform() method:
idx = df.groupby(['Sp', 'Mt'])['count'].transform(max) == df['count']
This will create a boolean Series where True indicates rows with maximum count.
Step 3: Filter Rows Based on Maximum Count
Finally, filter the DataFrame using the boolean indexing to select only the rows with maximum count:
result = df[idx]
This will return a new DataFrame containing only the rows with the highest value for the 'count' column within each group.
Examples:
Example 1:
df = pd.DataFrame({ 'Sp': ['MM1', 'MM1', 'MM1', 'MM2', 'MM2', 'MM2', 'MM4', 'MM4', 'MM4'], 'Mt': ['S1', 'S1', 'S3', 'S3', 'S4', 'S4', 'S2', 'S2', 'S2'], 'Value': ['a', 'n', 'cb', 'mk', 'bg', 'dgd', 'rd', 'cb', 'uyi'], 'count': [3, 2, 5, 8, 10, 1, 2, 2, 7] })
Output:
Sp Mt Value count 0 MM1 S1 a 3 2 MM1 S3 cb 5 3 MM2 S3 mk 8 4 MM2 S4 bg 10 8 MM4 S2 uyi 7
Example 2:
df = pd.DataFrame({ 'Sp': ['MM2', 'MM2', 'MM4', 'MM4', 'MM4'], 'Mt': ['S4', 'S4', 'S2', 'S2', 'S2'], 'Value': ['bg', 'dgd', 'rd', 'cb', 'uyi'], 'count': [10, 1, 2, 8, 8] })
Output:
Sp Mt Value count 4 MM2 S4 bg 10 7 MM4 S2 cb 8 8 MM4 S2 uyi 8
Note: If multiple rows within a group have the maximum count, all of those rows will be returned.
The above is the detailed content of How to Find Rows with the Maximum Count in Pandas GroupBy Operations?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










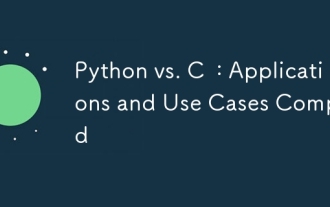
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
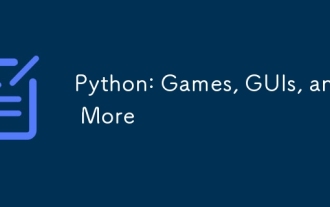
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
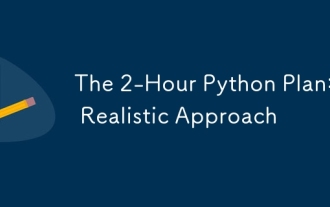
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
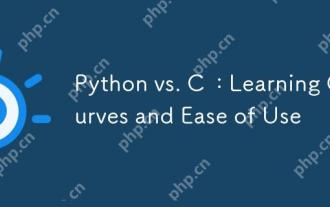
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
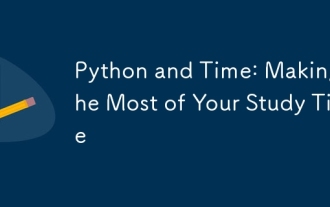
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
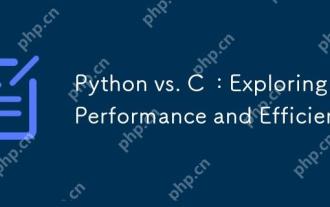
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
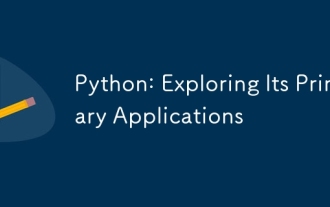
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
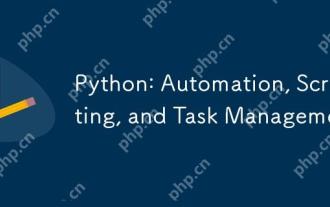
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
