


How Can I Write Generic Functions in Go to Handle Any Numerical Type?
Writing Generic Functions for Any Numerical Type in Go
When writing Go programs, you may encounter the need to create functions that can accept any numerical type, regardless of whether it's an integer or float. This can be challenging as Go strictly differentiates between these types.
Conversion to Float
One approach is to convert all numerical arguments to float64 since this type covers the range of integers. However, this may not always be desirable, as it may introduce precision loss or errors when dealing with large integers.
Separate Functions for Different Types
Another option is to create separate functions for each numerical type, such as addInt for integers and addFloat for floats. While this ensures type safety, it can lead to code duplication and maintenance issues.
Generics Using Type Parameters (Go 1.18 and above)
With the introduction of type parameters in Go 1.18, a more elegant solution is now available. You can define generic functions that take any type T and use interface constraints to restrict T to numeric types.
For example:
func add[T Number](a, b T) T { return a + b } type Number interface { constraints.Integer | constraints.Float }
This function can be used with any numeric type, including int, int64, float32, and float64. The Number constraint ensures that only numeric types are allowed.
Limitations
Keep in mind that generic functions require arguments of the same type. For example, the add function requires both arguments to be the same numeric type. Additionally, generic functions do not support all operators, particularly those specific to certain types (e.g., bitwise operators for integers).
Conclusion
Using type parameters and interface constraints allows you to write generic functions that can accept any numerical type. This approach provides type safety while avoiding code duplication and offering greater flexibility.
The above is the detailed content of How Can I Write Generic Functions in Go to Handle Any Numerical Type?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
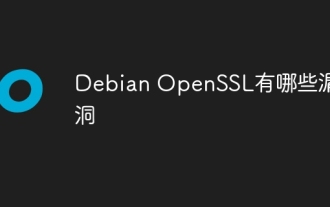
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
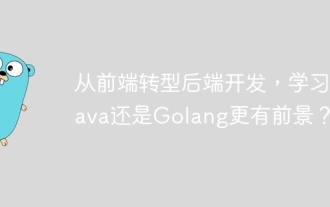
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
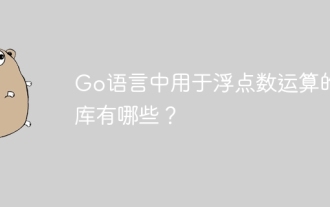
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
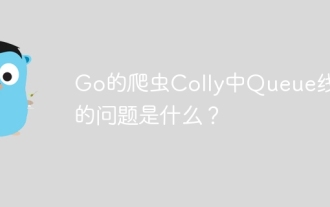
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
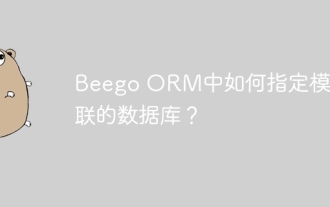
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
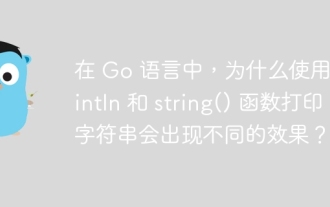
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
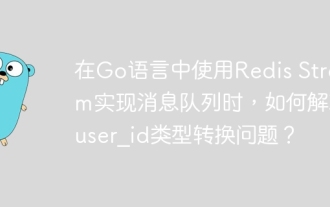
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
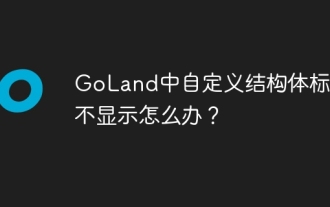
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
