How Can I Efficiently Copy Text to the Clipboard Using JavaScript?
How to Copy to the Clipboard in JavaScript
In JavaScript, there are multiple ways to copy text to the clipboard, depending on the browser support.
1. Async Clipboard API [navigator.clipboard.writeText]
Available in Chrome 66, the Async Clipboard API offers asynchronous access to the clipboard. It uses JavaScript Promises, allowing seamless execution without user prompts interrupting the page. Additionally, text can be directly copied to the clipboard from a variable. Note that it only works on pages served over HTTPS.
2. document.execCommand('copy') (Deprecated)
This method is synchronous and supported by most browsers since April 2015. It stops JavaScript execution until the process is complete, potentially displaying security prompts to the user. Text is copied from the DOM to the clipboard.
General Development Notes:
- Clipboard commands may not work while testing code in the console.
- Pages must be active (for Async Clipboard API) or require user interaction (for document.execCommand('copy')) to access the clipboard.
Fallback Method:
To ensure cross-browser compatibility, it's recommended to use a fallback method alongside the Async Clipboard API. Here's an example:
function fallbackCopyTextToClipboard(text) { // Create a textarea element and copy text to it const textArea = document.createElement("textarea"); textArea.value = text; // Append the textarea to the body and set properties document.body.appendChild(textArea); textArea.focus(); textArea.select(); // Execute the copy command try { var successful = document.execCommand('copy'); var msg = successful ? 'successful' : 'unsuccessful'; console.log('Fallback: Copying text command was ' + msg); } catch (err) { console.error('Fallback: Oops, unable to copy', err); } // Remove the textarea from the body document.body.removeChild(textArea); } function copyTextToClipboard(text) { if (!navigator.clipboard) { fallbackCopyTextToClipboard(text); return; } navigator.clipboard.writeText(text).then(function() { console.log('Async: Copying to clipboard was successful!'); }, function(err) { console.error('Async: Could not copy text: ', err); }); }
The above is the detailed content of How Can I Efficiently Copy Text to the Clipboard Using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
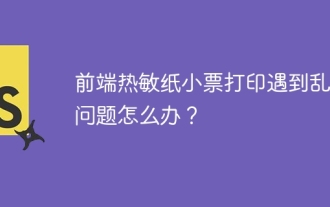
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
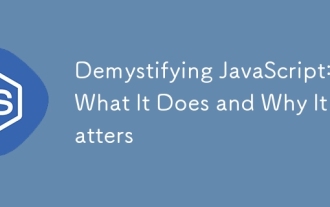
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
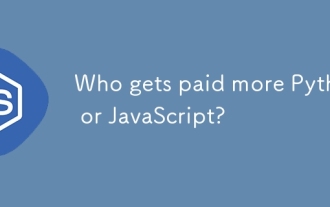
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
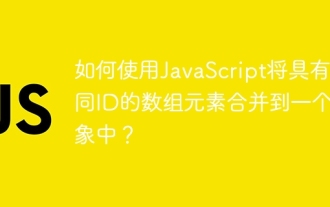
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
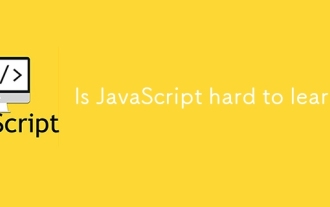
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
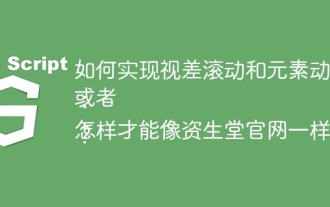
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
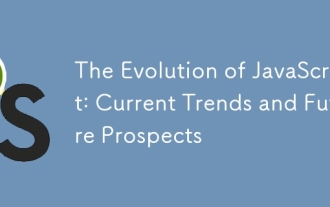
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
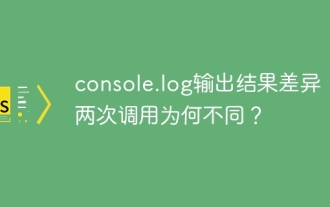
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
