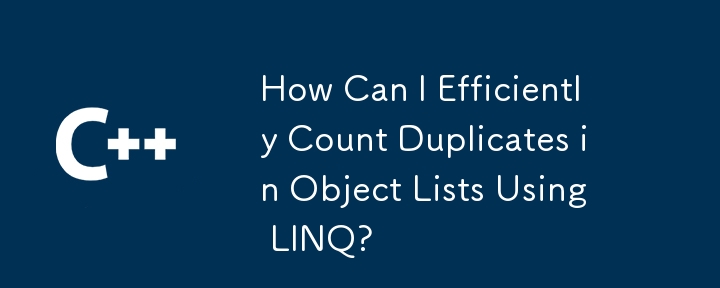
Counting Duplicates in Object Lists with LINQ
When working with lists of complex objects, counting duplicates can be a common requirement. Using LINQ (Language Integrated Query), you can efficiently group objects based on a common property and retrieve counts of each duplicate.
Here's a step-by-step guide on how to achieve this:
-
Group by Property: Use the GroupBy operator to group objects by the desired property. In the example provided, we want to count duplicates based on the "ID" property, so we can write:
var groupedIDs = items.GroupBy(item => item.ID);
Copy after login
-
Count Duplicates: For each group created, use the Count operator to determine the number of duplicate occurrences.
var duplicateCounts = groupedIDs.Select(group => new { ID = group.Key, Count = group.Count() });
Copy after login
-
Order Results: By default, the results will be grouped in arbitrary order. To sort by the highest number of duplicates, use the OrderByDescending operator:
var sortedDuplicates = duplicateCounts.OrderByDescending(x => x.Count);
Copy after login
-
Retrieve Selected Data: If you need both the count and the original object, you can use a projection in the Select operator to create a custom result:
var result = sortedDuplicates.Select(x => new { Count = x.Count, Object = x.ID });
Copy after login
-
Bind to Data Source: Once you have the duplicate counts, you can bind them to a data source for display or further processing. In your case, using ListView, this would look something like this:
lvRelatedGames.DataSource = result;
lvRelatedGames.DataBind();
Copy after login
Additional Considerations:
- If objects have different properties within each duplicate group, you may need to use a custom comparer to ensure that objects are considered duplicates based on specific criteria.
- The code provided is intended to be a starting point, and you may need to adapt it based on your specific requirements and data structure.
The above is the detailed content of How Can I Efficiently Count Duplicates in Object Lists Using LINQ?. For more information, please follow other related articles on the PHP Chinese website!