


How Does Java's Array Assignment Syntax Differ Inside and Outside of Declarations?
Array Assignment Syntax Outside of Declarations
Java's array initialization syntax differs depending on whether it's used in a declaration or an assignment.
Initializing with Array Literals
When declaring an array, array literals can be used to initialize its elements:
1 |
|
Initializing with the new Operator
Outside a declaration, arrays can be initialized using the new operator, followed by the element count and subsequent assignments:
1 2 3 |
|
Syntax Restrictions: Assigning Array Literals to Non-Declared Arrays
However, attempting to assign an array literal to a non-declared array variable is prohibited:
1 2 3 |
|
This restriction is imposed by Java for reasons that may not be immediately apparent.
Workaround: Using new Operator with Array Literals
As a workaround, the following syntax can be used:
1 2 3 |
|
This approach combines the array creation and initialization into a single statement while adhering to Java's syntax rules.
Example
For example, consider the following code snippet:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
|
Without the workaround, the code would require additional statements to initialize tempTab before assigning it to tab.
The above is the detailed content of How Does Java's Array Assignment Syntax Differ Inside and Outside of Declarations?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
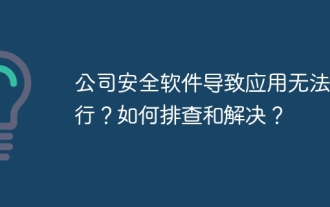
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
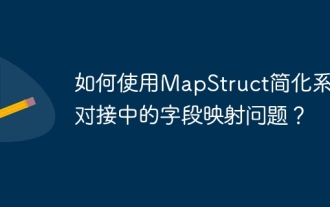
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
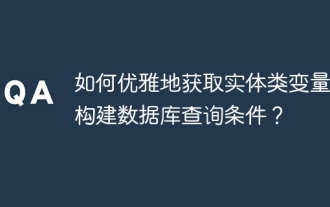
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
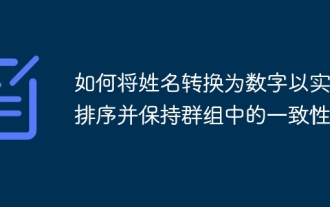
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
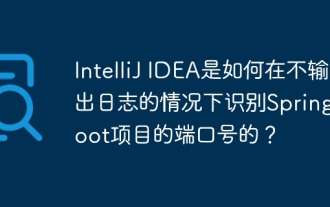
Start Spring using IntelliJIDEAUltimate version...
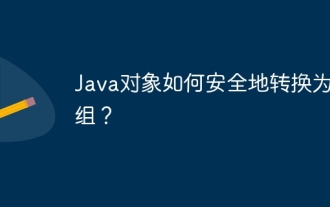
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
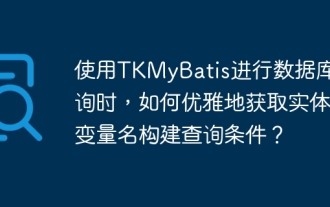
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
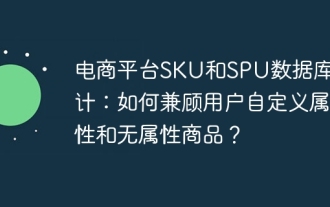
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
