How Should InterruptedExceptions Be Handled in Java?
Handling Interruptions in Java: Two Approaches and Best Practices
In Java, handling the InterruptedException is crucial when dealing with methods that can throw this exception. Two common approaches for handling this interruption are:
Propagating the Interruption (First Approach)
try { // ... } catch (InterruptedException e) { Thread.currentThread().interrupt(); }
This approach is suitable when the InterruptedException represents a valid outcome of the method and should be propagated to the caller. For instance, if a method performs a blocking network call that throws InterruptedException, it makes sense to let this exception propagate, indicating that the operation was interrupted.
Catching and Setting the Interrupted Flag (Second Approach)
try { //... } catch (InterruptedException e) { Thread.currentThread().interrupt(); throw new RuntimeException(e); }
This approach is preferred when the method cannot produce a valid result in case of interruption and should terminate. By setting the interrupted flag (Thread.currentThread().interrupt()), it ensures that the thread remains interrupted, informing the caller of the interruption.
Deciding the Best Approach
The appropriate approach depends on the situation:
- Propagating the Exception: If it makes sense for the method to allow for interruption and a normal return value cannot be produced (e.g., blocking network call), declare the method with throws InterruptedException and let the exception propagate.
- Catching and Setting the Interrupted Flag: If the method cannot complete the operation or return a valid result, catch the exception, set the interrupted flag, and throw a runtime exception.
Example (Second Approach)
void printSum(Server server) { try { int sum = computeSum(server); System.out.println("Sum: " + sum); } catch (InterruptedException e) { Thread.currentThread().interrupt(); // set interrupt flag System.out.println("Failed to compute sum"); } }
Here, the printSum method cannot complete its operation if interrupted, so it catches the InterruptedException, sets the interrupt flag, and prints an alternative message.
The above is the detailed content of How Should InterruptedExceptions Be Handled in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
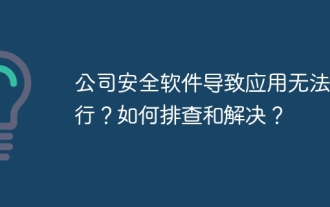
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
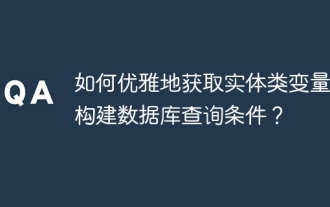
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
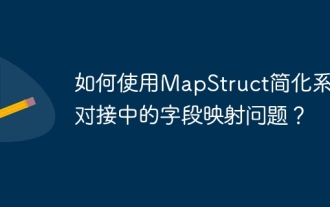
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
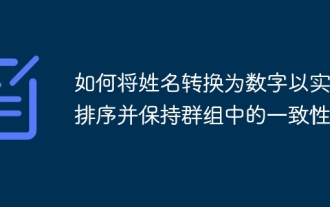
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
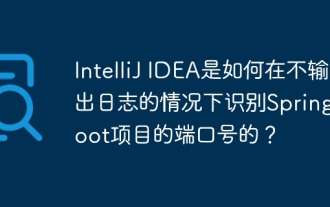
Start Spring using IntelliJIDEAUltimate version...
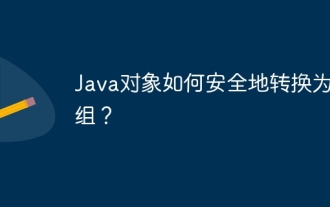
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
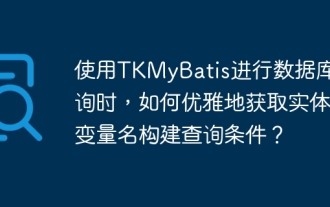
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
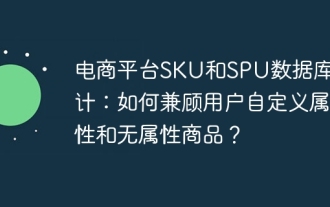
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
