Building a Golang Telegram Bot for Personal AI Assistance
Creating a Telegram bot that integrates with an AI assistant is an exciting project that combines real-time messaging, AI processing, and Golang's powerful concurrency model. In this blog, I'll guide you through the architecture, features, and implementation of a Telegram bot using Golang, with a complete system design to boot!
Overview
This project builds a personal AI assistant accessible via two interfaces:
Telegram Bot: A real-time conversational interface.
Console Chat: A terminal-based chatbot for direct interaction.
Key Features:
AI model switching based on user input.
Persistent chat history across sessions.
Interactive bot responses with live editing.
Retry mechanism for robust API handling.
System Design
Architecture
The system comprises the following components:
Bot Interface: Handles incoming messages, processes user input, and sends responses.
Assistant Utilities: Contains AI model integration logic.
History Management: Manages conversation history for persistence.
Error Handling: Ensures graceful error recovery.
Flow Diagram
[User] <---> [Telegram API] <---> [Bot API Handler] <---> [AI Processing Logic] ^ | | v [History Management] [Error Handler]
Components
- Telegram Bot API: Utilized for real-time messaging and updates.
- Golang’s Concurrency: To handle multiple user conversations seamlessly.
- History Module: Saves chat history in local files for reloadable sessions.
- Assistant Utilities: Processes user input and integrates AI logic.
Key Code Walkthrough
- Main Application Entry The main() function provides users with two modes: Telegram Bot and Console Chat. This ensures the assistant is accessible both online and offline.
func main() { fmt.Println("Choose mode: [1] Telegram Bot, [2] Console Chat") var choice int fmt.Scan(&choice) switch choice { case 1: deploy.TelegramBot() case 2: runConsoleChat() default: fmt.Println("Invalid choice.") } }
- Telegram Bot Initialization The bot uses go-telegram-bot-api for interacting with Telegram.
func NewTelegramBot() { token := os.Getenv("TELEGRAM_BOT_TOKEN") bot, err := NewBot(token) if err != nil { log.Fatal("Failed to start bot:", err) } bot.Start(context.Background()) }
- Handling User Messages
This method manages user interactions, including history loading and AI response handling.
[User] <---> [Telegram API] <---> [Bot API Handler] <---> [AI Processing Logic] ^ | | v [History Management] [Error Handler]
- Persistent History Management Chat history is managed through file-based persistence for continuity between sessions.
func main() { fmt.Println("Choose mode: [1] Telegram Bot, [2] Console Chat") var choice int fmt.Scan(&choice) switch choice { case 1: deploy.TelegramBot() case 2: runConsoleChat() default: fmt.Println("Invalid choice.") } }
- AI Processing Logic The bot integrates with an AI assistant, handling retries and partial responses.
func NewTelegramBot() { token := os.Getenv("TELEGRAM_BOT_TOKEN") bot, err := NewBot(token) if err != nil { log.Fatal("Failed to start bot:", err) } bot.Start(context.Background()) }
Want a demo ?

Building a Golang Telegram Bot for Personal AI Assistance | by Mukul Saini | Dec, 2024 | Medium
Mukul Saini ・ ・
Medium

demo
Conclusion
With this bot, we leverage Golang's concurrency and efficient libraries to build a scalable and interactive AI assistant. The integration with Telegram API ensures a seamless real-time experience for users. Start building yours today and explore the power of AI-driven conversations!
The above is the detailed content of Building a Golang Telegram Bot for Personal AI Assistance. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










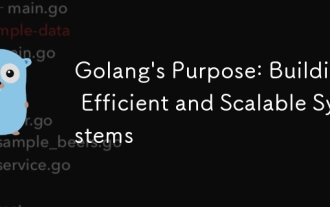
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
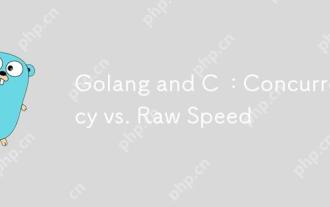
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
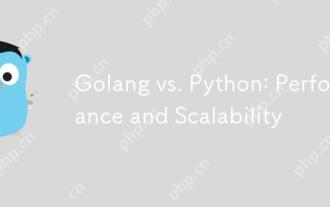
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
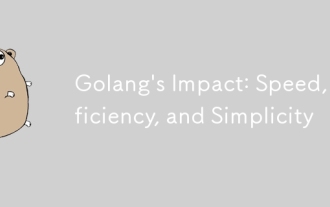
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
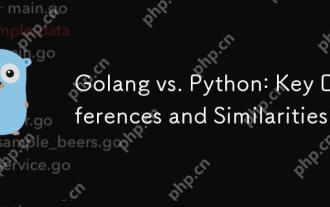
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
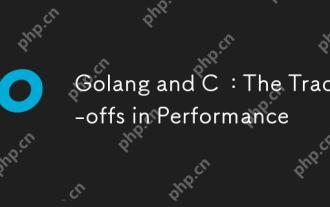
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
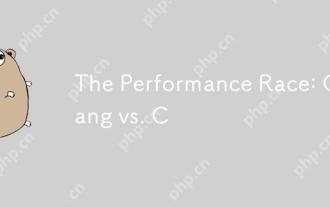
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
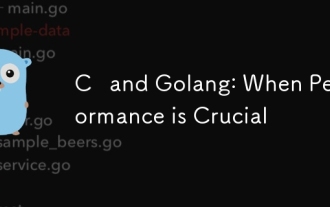
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
