How Can I Efficiently Find Unique Rows in a NumPy Array?
Finding Unique Rows in a NumPy Array: An Efficient Solution
In data analysis and processing, it is often necessary to extract unique values from a given dataset. In this context, let's consider the problem of finding unique rows in a NumPy array.
Objective:
Given a NumPy array, the goal is to identify and obtain an array containing only the unique rows in the original array.
Efficient Solution:
As of NumPy version 1.13, an efficient solution for finding unique rows has been introduced. By leveraging the np.unique function and specifying the axis parameter, we can achieve this with ease:
unique_rows = np.unique(original_array, axis=0)
By setting the axis parameter to 0, we instruct NumPy to analyze each row of the original array independently. This operation compares the rows element-wise and returns a new array that contains only the unique rows.
Example:
Consider the following NumPy array a:
a = np.array([[1, 1, 1, 0, 0, 0], [0, 1, 1, 1, 0, 0], [0, 1, 1, 1, 0, 0], [1, 1, 1, 0, 0, 0], [1, 1, 1, 1, 1, 0]])
To obtain the unique rows from a, we can use the following code:
unique_rows = np.unique(a, axis=0)
This will produce a new array unique_rows that contains the following rows:
unique_rows = np.array([[1, 1, 1, 0, 0, 0], [0, 1, 1, 1, 0, 0], [1, 1, 1, 1, 1, 0]])
As we can see, the unique rows in the original array have been successfully extracted.
The above is the detailed content of How Can I Efficiently Find Unique Rows in a NumPy Array?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
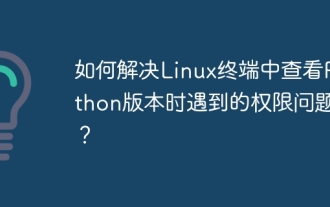
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
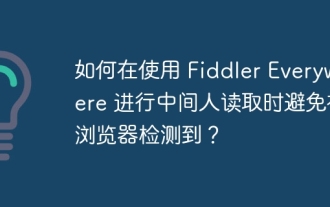
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
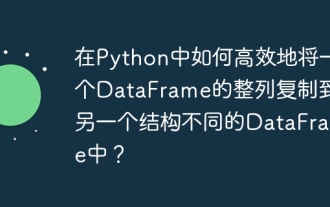
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
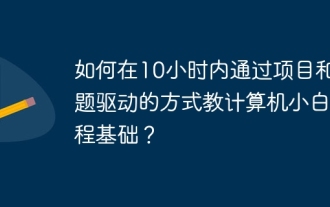
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
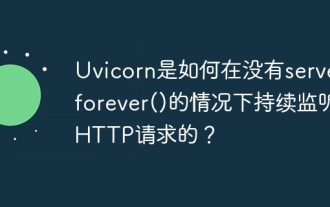
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
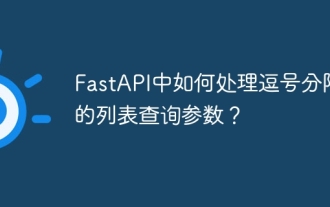
Fastapi ...
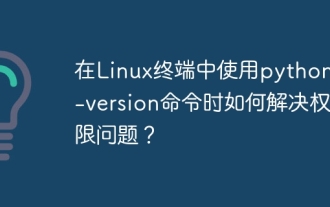
Using python in Linux terminal...
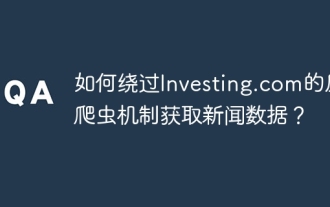
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
