


How to Handle Null Values When Unmarshalling MongoDB Documents in Go?
Handling Null Values While Unmarshalling MongoDB Documents
Ignoring null values during the unmarshalling process of MongoDB documents into Go structs is a common need. In this article, we'll explore a solution using custom decoders in the mongo-go-driver.
Custom String Decoder
Initially, let's handle null values for strings. We define a custom decoder that interprets null values as empty strings:
import ( "go.mongodb.org/mongo-driver/bson/bsoncodec" "go.mongodb.org/mongo-driver/bson/bsonrw" ) type nullawareStrDecoder struct{} func (nullawareStrDecoder) DecodeValue(dctx bsoncodec.DecodeContext, vr bsonrw.ValueReader, val reflect.Value) error { if !val.CanSet() || val.Kind() != reflect.String { return errors.New("bad type or not settable") } var str string switch vr.Type() { case bsontype.String: str, _ = vr.ReadString() case bsontype.Null: _ = vr.ReadNull() default: return fmt.Errorf("cannot decode %v into a string type", vr.Type()) } val.SetString(str) return nil }
Utilizing Custom Decoder
To use this custom decoder for a specific client, we register it with the client's registry:
clientOpts := options.Client(). ApplyURI("mongodb://localhost:27017/"). SetRegistry( bson.NewRegistryBuilder(). RegisterDecoder(reflect.TypeOf(""), nullawareStrDecoder{}). Build(), ) client, err := mongo.Connect(ctx, clientOpts)
Type-Neutral Decoder for Any Null Value
A more comprehensive approach involves creating a type-neutral decoder that handles null values for any type:
import ( "go.mongodb.org/mongo-driver/bson/bsoncodec" "go.mongodb.org/mongo-driver/bson/bsonrw" "reflect" ) type nullawareDecoder struct { defDecoder bsoncodec.ValueDecoder zeroValue reflect.Value } func (d *nullawareDecoder) DecodeValue(dctx bsoncodec.DecodeContext, vr bsonrw.ValueReader, val reflect.Value) error { if vr.Type() != bsontype.Null { return d.defDecoder.DecodeValue(dctx, vr, val) } if !val.CanSet() { return errors.New("value not settable") } _ = vr.ReadNull() val.Set(d.zeroValue) return nil }
For each type we want nulls to be ignored for, we can use this decoder. For example, for strings and integers:
customValues := []interface{}{ "", // string int(0), // int } rb := bson.NewRegistryBuilder() for _, v := range customValues { t := reflect.TypeOf(v) defDecoder, err := bson.DefaultRegistry.LookupDecoder(t) if err != nil { panic(err) } rb.RegisterDecoder(t, &nullawareDecoder{defDecoder, reflect.Zero(t)}) } clientOpts := options.Client(). ApplyURI("mongodb://localhost:27017/"). SetRegistry(rb.Build()) client, err := mongo.Connect(ctx, clientOpts)
With this setup, values for the specified types will be set to their respective zero values when nulls are encountered during unmarshalling.
The above is the detailed content of How to Handle Null Values When Unmarshalling MongoDB Documents in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
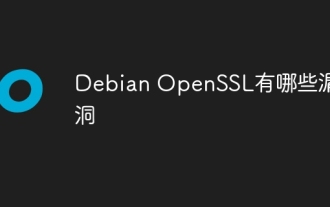
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
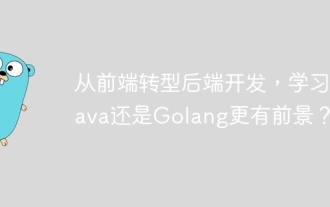
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
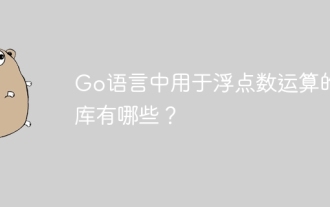
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
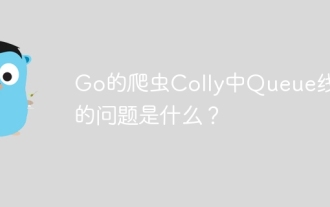
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
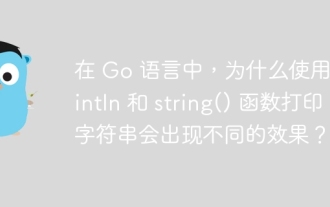
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
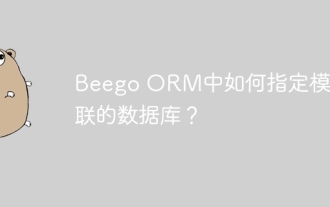
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
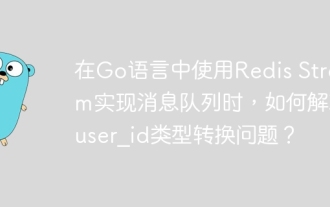
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
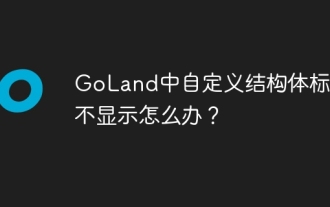
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
