How to Sort a Go Map by its Integer Values?
Sorting a Map by Value in Go
Given a map with string keys and integer values, we may encounter the need to sort the map by its values in a specific order. This tutorial outlines a solution to this common programming task.
Solution
One approach to sorting a map by value in Go is to create a custom data structure that implements the sort.Interface. This interface defines the Len, Less, and Swap methods, which are used by Go's sorting algorithms to determine the order of elements.
Here's an example code block that demonstrates the implementation:
// RankByWordCount sorts a map[string]int by its values in descending order. func rankByWordCount(wordFrequencies map[string]int) PairList { pl := make(PairList, len(wordFrequencies)) i := 0 for k, v := range wordFrequencies { pl[i] = Pair{k, v} i++ } sort.Sort(sort.Reverse(pl)) return pl } // Pair represents a key-value pair. type Pair struct { Key string Value int } // PairList is a list of Pair. type PairList []Pair // Len returns the length of the PairList. func (p PairList) Len() int { return len(p) } // Less compares two Pair and returns true if the first one should be // placed after the second one in the sorted list. func (p PairList) Less(i, j int) bool { return p[i].Value < p[j].Value } // Swap swaps two elements in the PairList. func (p PairList) Swap(i, j int){ p[i], p[j] = p[j], p[i] }
In the provided code, rankByWordCount takes a map[string]int as input and creates a PairList which contains key-value pairs sorted in descending order based on the values. It utilizes Go's built-in sorting algorithms to perform the sorting.
To use this function, you can provide your map as the input and obtain a PairList of sorted key-value pairs.
Remember to import the sort package in your code to use these sorting functions effectively.
The above is the detailed content of How to Sort a Go Map by its Integer Values?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


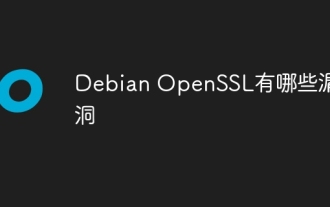
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
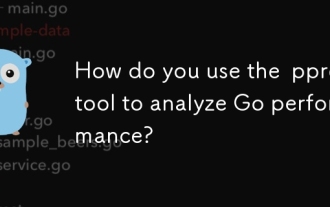
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
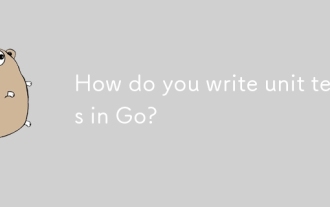
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
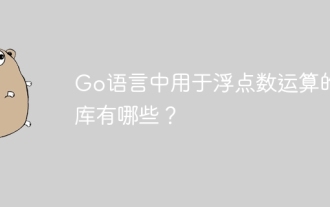
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
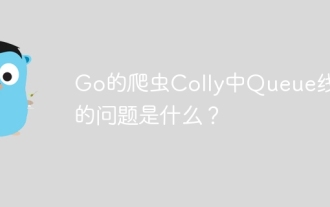
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
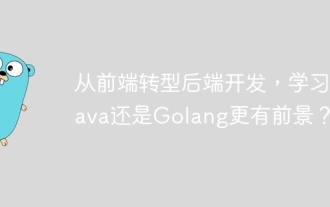
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
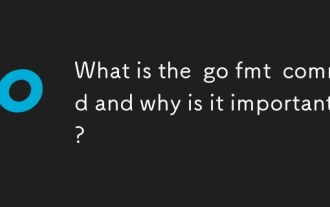
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
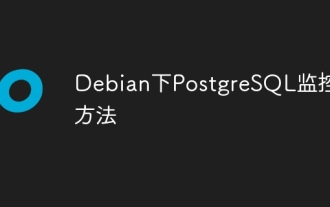
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
