


How Does Python's `super()` Function Work with Multiple Inheritance and Method Resolution Order (MRO)?
Python's super() and Multiple Inheritance
In Python, multiple inheritance introduces complexities when determining which parent method to invoke. The super() function plays a crucial role in this scenario, but its behavior depends on the method resolution order (MRO).
MRO and super()
The MRO defines the sequence in which parent classes are searched for attributes. In your example:
class Third(First, Second): def __init__(self): super(Third, self).__init__() print "that's it"
super(Third, self).__init__() calls the __init__() method of the first class in the MRO. In this case, it's First.
Choice of Parent Method
You cannot explicitly choose which parent method to call using super(). The MRO dictates the order of search, and super() always refers to the first class in that order.
Example (Single Path Inheritance)
In your example with no crossed inheritance, the MRO is straightforward: [Third, First, Second, object]. Therefore, super(Third, self).__init__() will always call First.__init__().
Example (Crossed Inheritance)
Consider the following code:
class First(object): def __init__(self): print "first" class Second(First): def __init__(self): print "second" class Third(First): def __init__(self): print "third" class Fourth(Second, Third): def __init__(self): super(Fourth, self).__init__() print "that's it"
The MRO is now [Fourth, Second, Third, First, object]. super(Fourth, self).__init__() will call Second.__init__(), the first class in the MRO.
Ambiguous MRO
If the inheritance paths交叉 (e.g., First inherited from Second), Python cannot establish a coherent MRO, resulting in an exception:
TypeError: Cannot create a consistent method resolution order (MRO) for bases Second, First
Conclusion
super() is a powerful tool for working with multiple inheritance. However, understanding the MRO is crucial to predicting its behavior, as it dictates which parent method will be invoked when using super().__init__().
The above is the detailed content of How Does Python's `super()` Function Work with Multiple Inheritance and Method Resolution Order (MRO)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










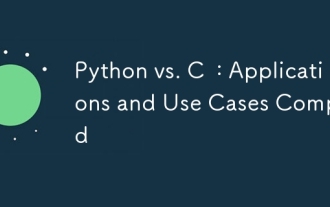
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
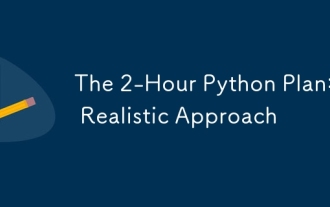
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
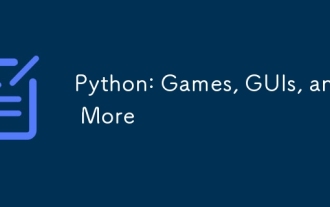
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
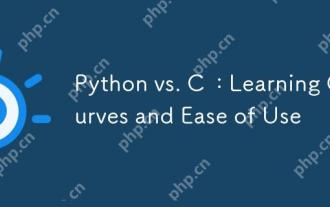
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
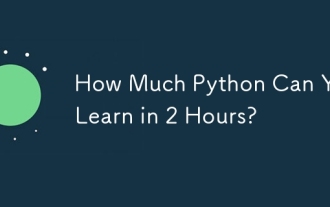
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
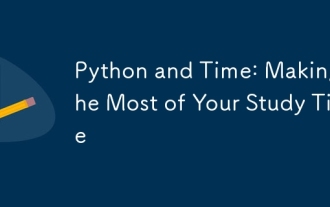
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
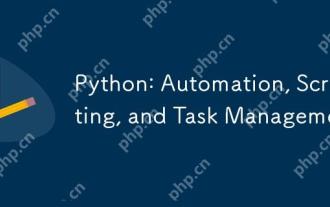
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
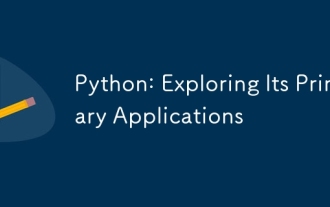
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
