


How to Alphabetize Strings with Embedded Numbers While Preserving Numerical Order?
Dec 30, 2024 am 10:16 AMHow to Sort Strings Alphabetically While Preserving Numeric Values
Sorting strings alphabetically can encounter challenges when some of the strings contain numeric characters. If numeric conversion is prohibited, an alternative approach is required to handle these cases effectively.
One solution is to utilize a custom comparer that distinguishes between numeric and non-numeric values. This comparer can be passed to the OrderBy method to customize the sorting criteria.
Here's an example implementation using the Enumerable.OrderBy method and a SemiNumericComparer class:
using System; using System.Collections.Generic; class Program { static void Main() { string[] things = new string[] { "paul", "bob", "lauren", "007", "90", "101" }; foreach (var thing in things.OrderBy(x => x, new SemiNumericComparer())) { Console.WriteLine(thing); } } public class SemiNumericComparer: IComparer<string> { /// <summary> /// Determine if a string is a number /// </summary> /// <param name="value">String to test</param> /// <returns>True if numeric</returns> public static bool IsNumeric(string value) { return int.TryParse(value, out _); } /// <inheritdoc /> public int Compare(string s1, string s2) { // Flags to indicate if strings are numeric var IsNumeric1 = IsNumeric(s1); var IsNumeric2 = IsNumeric(s2); // Handle numeric comparisons if (IsNumeric1 && IsNumeric2) { var i1 = Convert.ToInt32(s1); var i2 = Convert.ToInt32(s2); // Order by numerical value if (i1 > i2) { return 1; } if (i1 < i2) { return -1; } return 0; } // Handle cases where only one string is numeric if (IsNumeric1) { return -1; } if (IsNumeric2) { return 1; } // Default to alphabetical comparison return string.Compare(s1, s2, true, CultureInfo.InvariantCulture); } } }
This code will output the strings in the following sorted order:
007 90 bob lauren paul 101
The above is the detailed content of How to Alphabetize Strings with Embedded Numbers While Preserving Numerical Order?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
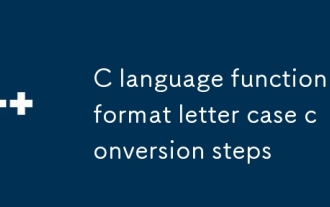
C language function format letter case conversion steps
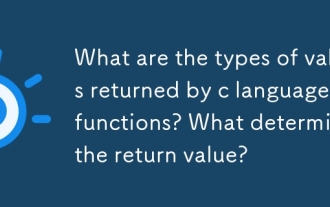
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
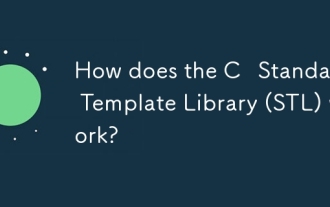
How does the C Standard Template Library (STL) work?

Where is the return value of the c language function stored in memory?
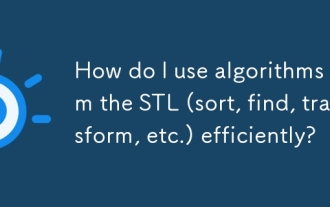
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
