


How Can I Work Around Go's JSON Tag Restrictions Using Unexported Structs and Type Casting?
Using Type Casting within Unexported Structs
In Go, you can't define multiple JSON tags for the same field in a struct. However, there is a workaround using casting between unexported structs.
First, create two identically-structured structs with different JSON tags, as in your example:
type Foo struct { Name string `json:"name"` Age int `json:"age"` } type Bar struct { Name string `json:"employee_name"` // Age omitted with backtick syntax Age int `json:"-"` }
Now, make Bar unexported by starting its name with a lowercase letter:
type bar struct { Name string `json:"employee_name"` Age int `json:"-"` }
To transform from Foo to bar, cast Foo to *bar, as shown below:
f := Foo{Name: "Sam", Age: 20} b := (*bar)(unsafe.Pointer(&f)) // b now has the modified JSON tags
Cautions:
- This technique should be used cautiously and only within a controlled environment.
- Never expose the unexported bar type outside of the current package.
- Ensure that the types are always aligned, otherwise casting will panic.
Example:
package main import "fmt" import "unsafe" type Foo struct { Name string `json:"name"` Age int `json:"age"` } type bar struct { Name string `json:"employee_name"` Age int `json:"-"` } func main() { f := Foo{Name: "Sam", Age: 20} b := (*bar)(unsafe.Pointer(&f)) fmt.Println(b.Name, b.Age) // Output: Sam 0 // Changing f.Age affects b.Age f.Age = 30 fmt.Println(b.Name, b.Age) // Output: Sam 30 }
The above is the detailed content of How Can I Work Around Go's JSON Tag Restrictions Using Unexported Structs and Type Casting?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










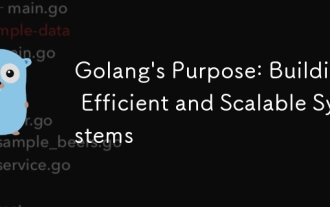
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
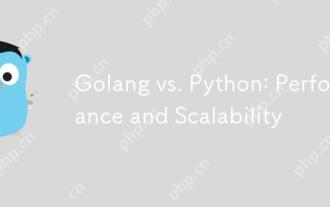
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
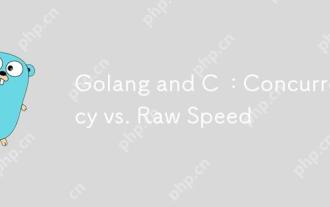
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
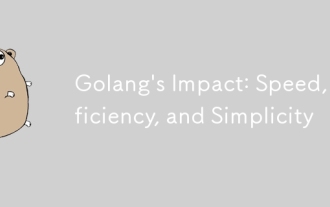
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
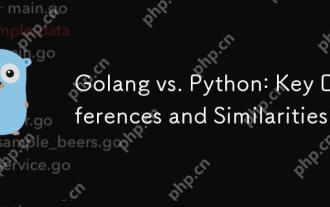
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
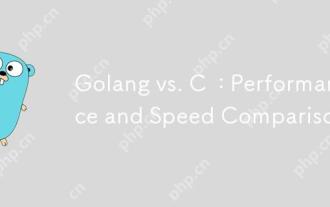
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
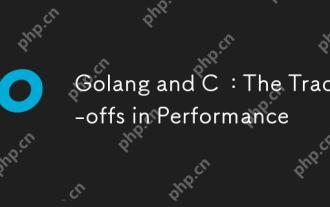
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
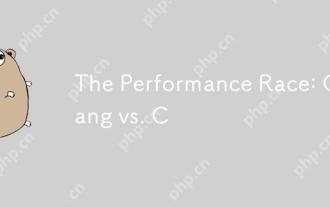
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
